Sending email reliably is crucial for any web application. However, between transient Gmail queues, Nodemailer connection issues, throttling limits, and achieving deliverability at scale – it can quickly become a headache. In this comprehensive guide, we dive into troubleshooting common Gmail and Node.js email delivery problems. Learn how Gmail’s internal queuing and throttling work, optimize Nodemailer to avoid errors like ECONNREFUSED, handle issues with services like Mailgun, validate addresses to improve deliverability, and leverage tools like SES and Mailgen for large volume sending. With proactive performance monitoring and capacity planning, you can confidently send email from your Node apps at any volume without deliverability mishaps. The road to inbox success begins here.
When sending emails through Gmail, you may occasionally see a message with a status of “queued.” This means Gmail has accepted the message but has not yet attempted to deliver it to the recipient’s inbox. Understanding Gmail’s queuing system can help troubleshoot cases where your messages get stuck in the queue.
Overview of Gmail’s Queued Message System
Gmail does not immediately send every email you compose. Instead, it uses a queued delivery system to optimize performance and deliverability.
When you hit send, Gmail accepts your email into its outgoing message queue. This queue acts as a temporary holding area for all pending emails. Gmail then works through this queue, attempting to deliver each message based on its internal priority algorithm.
Some key points about Gmail’s queuing system:
- Emails typically spend just a few minutes in the queue before send attempts begin. But queues can backup during peak traffic periods.
- Gmail throttles how quickly it delivers messages from the queue to protect its IP reputation and avoid overloading recipients’ inboxes.
- Prioritization factors include message size, recipient domains, attachment types, and more.
- Messages that fail to send enter the retry queue for additional attempts over the next few hours.
So in summary – the “queued” status simply means your message is awaiting its turn to be transmitted based on Gmail’s batched sending protocols.
Reasons an Email May Get Stuck in Gmail’s Queue
Under normal circumstances, an email spends just a short time in Gmail’s outbound message queue before delivery begins. But occasionally, a message can get stuck here and delayed far longer than expected.
Some common reasons an email may linger in Gmail’s queue include:
- Temporary capacity limits – During periods of high traffic, Gmail’s queue capacity fills up. Newer messages will remain queued until space frees up.
- ** Recipient throttling** – If Gmail detects spammy behavior toward a domain, it will throttle send rates, delaying delivery.
- Attachment filters – Messages with certain attachment types like .exe and .zip files require additional scanning before send.
- Priority deprioritization – Bulk messages or those with many recipients may be deprioritized, keeping them queued longer.
- ** Undisclosed recipients** – Gmail may delay messages sent to undisclosed recipients (BCC) to protect privacy.
- ** Routing issues** – Network interruptions when routing a message can require retries.
- ** Reputation-based throttling** – Gmail may throttle delivery to new recipients or domains with no send history.
- ** Spam filters** – Messages flagged as potential spam require additional checks before release.
So if an important email gets stuck in Gmail’s queue for an extended period, one of these factors likely came into play.
How Long Do Queued Gmail Messages Typically Take to Send?
For most standard emails, the queued status lasts just a few minutes. Gmail usually begins its first send attempt within 5 minutes of receiving your message.
However, queued delivery time frames can vary quite a bit depending on your message’s contents, recipients, and current traffic conditions:
- Basic text-only emails to known recipients normally send in under 5 minutes.
- Messages with large attachments often queue longer, taking 15+ minutes to begin sends.
- Delivery to new international domains you’ve never contacted before may be delayed up to several hours.
- Bulk emails with many different recipients tend to queue longer on average.
- During peak traffic times like the holidays, queues can become backlogged by several hours.
As a rule of thumb, if an email remains queued beyond 30-60 minutes with no send attempts, there is likely an underlying issue causing the delivery delay.
Troubleshooting Tips for Fixing Stuck Gmail Queued Messages
If an important outgoing message gets stuck in Gmail’s queue, here are some troubleshooting steps to force it through:
- Restart sending – Open the draft and hit send again. This re-queues the message, overriding any throttling.
- Remove attachments – Bulk attachments like images often delay sends. Try removing them.
- Use fewer recipients – Large recipient lists trigger extra scanning. Reduce the To/CC/BCC field size.
- Split into multiple emails – Smaller individual emails may bypass throttling triggers.
- Use plain text – Fancy formatting like images and attachments queue longer. Switch to plain text.
- Alter subject line – Spammy subject lines with ALL CAPS or special characters cause delays.
- Switch send account – Try sending from an alternate Gmail or provider account.
- Double check recipients – Incorrect addresses delay sends, verify them.
- Wait and retry – Temporary traffic jams often resolve within a few hours.
Following these tips should help troubleshoot and hopefully release your stuck message from Gmail’s outbound queue limbo. But if a message continues failing to send after 24 hours, it likely requires further investigation to identify the root cause.
Gmail’s queuing system allows it to optimize delivery speed and efficiency behind the scenes. While typically invisible to users, extended queued times can occasionally delay message transmission. Understanding the queue’s purpose along with causes and solutions for stuck messages makes troubleshooting easier when critical emails fail to send in a timely manner.
Sending email is a common need for many Node.js applications. However, handling email reliably can be tricky, especially when using a JavaScript framework like Express. Connection errors, blocked messages, and poor deliverability are just some of the potential pitfalls.
Fortunately, excellent Node.js packages exist like Nodemailer to abstract away much of the email complexity. With the right setup and some debugging knowledge, you can configure Nodemailer to send email smoothly without issues.
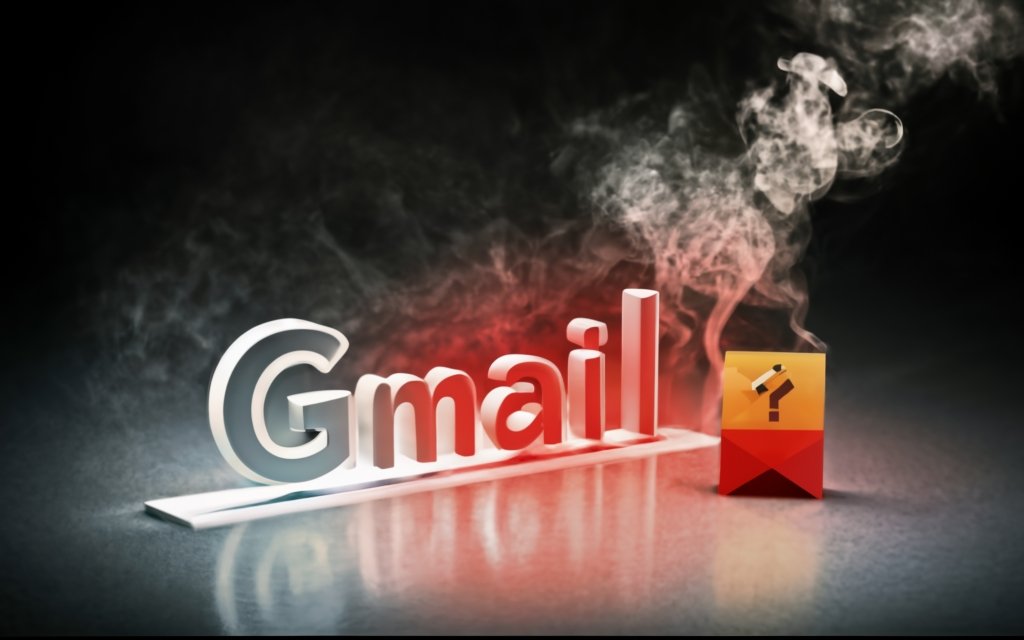
Common JS Email Packages Like Nodemailer and SendGrid
There are quite a few packages for sending email from Node, but two popular choices are:
- Nodemailer – A general purpose Node.js module for email. It handles creating connections as well as email formatting.
- SendGrid – A cloud-based email service with a Node.js library. It handles deliverability but requires a paid SendGrid account.
Nodemailer is the more flexible open-source option working with any email service. SendGrid is ideal for those already paying for its delivery infrastructure. Both integrate easily into Express apps.
To install either package:
// Install via npm
npm install nodemailer
npm install @sendgrid/mail
Then import in your Node.js code:
// Require the packages
const nodemailer = require('nodemailer');
const sgMail = require('@sendgrid/mail');
With just a few lines of code, you can begin sending email through existing SMTP servers or services like SendGrid.
Setting Up Nodemailer in Your Node.js App
To use Nodemailer, first create a “transporter” object configuring the email sending connection:
// Create Nodemailer transporter
let transporter = nodemailer.createTransport({
host: 'smtp.example.com',
port: 587,
auth: {
user: 'your_username',
pass: 'your_password'
}
});
This handles authenticating to an SMTP server for sending. Popular services like Gmail, Mailgun, and SendinBlue can also be configured here.
Then you can send messages using:
// Send email
let info = await transporter.sendMail({
from: '[email protected]',
to: '[email protected]',
subject: 'Nodemailer test',
text: 'Hello world!'
});
The sendMail() method queues the email for delivery. Callback based sending is also available.
With just a few lines, you’ve set up Nodemailer for sending email through a custom or third-party SMTP server from your Node.js app!
Testing and Troubleshooting Send Failures
When first integrating any email package, it’s critical to test and validate email sending before relying on it for production.
Some tips for testing and troubleshooting:
- Use your own email addresses first during testing so you can inspect delivery.
- Check spam folders as sometimes initial emails get flagged.
- Try sending with third-party services like Mailgun and SendGrid to diagnose issues.
- Log and inspect errors – they often indicate configuration problems.
- Double check username, password, port, and server settings entered.
- Check connectivity to the SMTP servers with tools like Telnet.
With Nodemailer itself, you can listen for the sent
and error
events:
// Monitor sent & error events
transporter.on('sent', () => {
console.log('Email was sent successfully!');
});
transporter.on('error', (err) => {
console.log('Error occurred sending email: ' + err);
});
This makes diagnosing problems in your Node.js app easier during development.
Handling Nodemailer Errors Like ECONNREFUSED
Once you begin sending larger volumes of email in production, you may encounter intermittent errors like:
Error: connect ECONNREFUSED x.x.x.x:465
This means the connection was refused when trying to connect to the configured SMTP server.
Some potential causes include:
- Incorrect SMTP host or port configured
- Network connectivity issues
- Throttling from your email service provider
- Incorrect SSL/TLS settings
- Temporary SMTP server problems
To further debug ECONNREFUSED errors:
- Verify the hostname, port and SSL settings entered.
- Check the network – can you ping the SMTP server’s IP?
- Try Telnet to test manually connecting on the port.
- Enable SMTP debugging output in Nodemailer to log connection data.
- Attempt sends using a different SMTP server to isolate the problem.
With consistent connection refused errors, using a dedicated third party delivery service like SendGrid or Mailgun can avoid ongoing reliability issues.
Best Practices for Reliable Nodemailer Email Sending
Beyond correct setup and troubleshooting issues, some Nodemailer best practices include:
- Use services like Mailgun or SparkPost until email volumes necessitate managing your own SMTP server.
- Enable SMTP connection pooling in Nodemailer for better performance.
- Implement queuing to smooth out traffic spikes and failures.
- Always validate recipient addresses before sending.
- Employ email address warming techniques to avoid spam folders.
- Set From addresses properly and configure SPF/DKIM records.
- Monitor sent & error events to catch problems early.
- Consider dedicated IP addresses to protect sender reputation.
- Test, test test before launching production email capabilities!
Adopting these practices avoids many deliverability and reliability pitfalls that can plague production email sending.
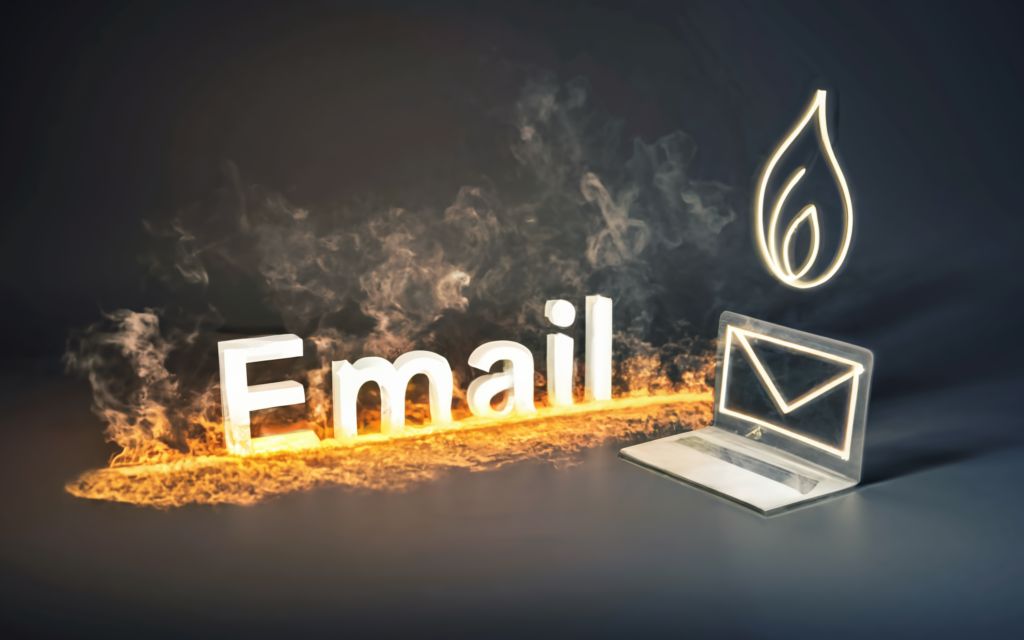
Fixing Nodemailer ECONNREFUSED Connection Timeouts
Connection refused and network timeout errors are common Nodemailer issues that frustrate developers.
Some strategies for avoiding timeout and ECONNREFUSED errors include:
Check Your SMTP Server Settings
- Confirm the hostname matches your SMTP server exactly.
- Validate the port number – common ports are 25, 465 or 587.
- Try toggling SSL/TLS settings if having TLS issues.
Test Basic Connectivity to the Server
- Can you ping the SMTP server’s IP from the host machine?
- Use Telnet to manually connect to the SMTP port as a test.
Retry Sending With a Different SMTP Server
- Sign up for a free Mailgun, SendinBlue or SparkPost account.
- Configure Nodemailer to use them as the SMTP server.
- Retry sending – if it works, your own server is likely misconfigured.
Increase Nodemailer Timeout Limits
- The default Nodemailer timeout is 5 seconds – increase it.
- Set
connectionTimeout
andsocketTimeout
options higher.
Following this methodology isolates connection issues down to server settings, network problems or Nodemailer configuration itself. Adjusting timeouts provides more leeway for send attempts.
Avoid Send Limit and Blocks With Services Like Mailgun
Another common issue when first sending large volumes of email is hitting usage limits and getting blocked by providers.
This is especially true with free tiers of email services like Mailgun, which impose daily sending limits. Even fully paid accounts have send bandwidth restrictions.
Understanding Mailgun and Other Provider Limits
Let’s look at Mailgun as an example – their free plan includes:
- Up to 300 emails per day
- No more than 3 emails per second
- Maximum 10,000 emails per month
Exceeding these limitations, such as sending 5,000 emails immediately, will trigger overage blocking until the next day.
Paid plans have higher limits, but still restraints like 50,000 emails per hour.
Techniques to Avoid Hitting Mailgun Limits
Here are some techniques to avoid triggering Mailgun’s limits:
- Queue emails and spread out delivery over time.
- Limit concurrent connection counts to respect 3 email/sec limit.
- Throttle individual recipients to 1 email per 15 min.
- Batch larger blasts into chunks under 10,000 per month.
- Warm up IP by sending increasing volumes over several days.
Following these best practices prevents spikes in traffic that exceed caps and get accounts suspended.
Fixing a Blocked Mailgun Account
If you do trigger an overage block with Mailgun, here are some recovery steps:
- Wait it out – Most blocks lift automatically after a day or two.
- Contact support – Mailgun support can lift some usage blocks manually.
- Transition to paid plan – Paid plans have higher limits, avoiding blocks.
- Switch providers – Try SendGrid, SparkPost or SES to reset limits.
With some adjustments to email patterns and traffic volume management, services like Mailgun become more reliable.
Other Email Services With Limits to Avoid
Mailgun isn’t the only provider with strict send limits – be mindful when evaluating other services as well:
- Amazon SES has complex monthly quotas once out of sandbox.
- SparkPost blocks at 500 emails per hour for free tiers.
- SendGrid limits you to 100 emails per second.
- SMTP2GO has a 250 email per hour limit on low tiers.
The key is gradually ramping up volumes while implementing throttling and queueing to avoid sudden traffic spikes.
In Summary
Configuring reliable production email delivery with Nodemailer does require some trial and error. However, by leveraging connection pooling, queuing, and reputable providers you can achieve great results sending email from your Node.js apps. Monitoring for errors, validating addresses, and testing diligently also helps smooth out the process.
Email sending is rarely flawless out of the gate. But with consistent performance tuning and capacity planning, the headaches around timeouts, blocks, and bounces can be diminished substantially. Give your users the fast, dependable email experience they deserve.
Sending email to invalid or non-existent addresses is all too common. These mistakes result in frustrating bounced messages and harm your sender reputation. Verifying email addresses before attempting delivery is crucial for avoiding deliverability issues.
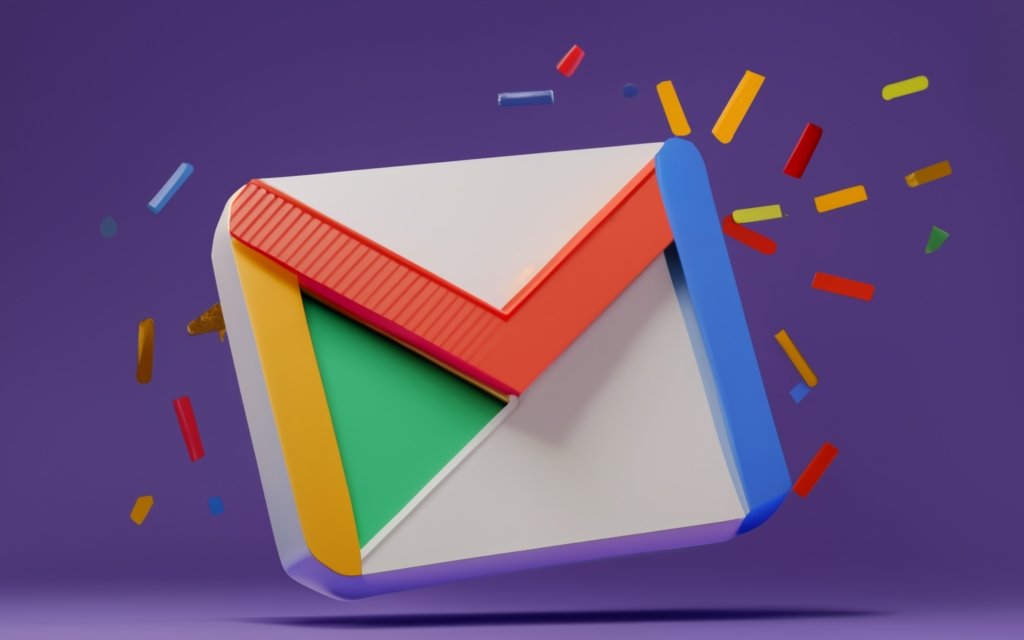
Why Invalid Email Addresses Cause Bounces
When you send an email to an address like [email protected] that doesn’t actually exist, the recipient mail server will reject the message with a bounce.
These hard bounces occur for reasons like:
- Recipient address literally does not exist or is deactivated.
- Domain name is invalid or misspelled.
- Mailbox has exceeded size limits.
Soft bounces happen when a valid mailbox is temporarily unavailable, often due to a full inbox or inactive account.
No matter the bounce type, they negatively impact your sender rating with major ISPs like Gmail. Too many bounces and you may find messages blocked as spam.
Verifying email validity before attempting a send prevents these useless failed deliveries.
Node.js Packages to Validate Addresses
To validate addresses in Node.js, two helpful packages are:
validator – A general input validation library with email checks:
const validator = require('validator');
const isValid = validator.isEmail('[email protected]');
email-validator – A dedicated email validation module:
const emailValidator = require('email-validator');
const {valid, reason} = emailValidator.validate('[email protected]')
Both can validate syntax and confirm if an address exists by connecting to the domain’s MX records.
For large lists, also consider domain validation in bulk vs one by one.
Adding Email Verification in Express
To implement email verification in an Express web app, add validation during:
Signup
// Validate on signup route
router.post('/signup', (req, res) => {
if (!validator.isEmail(req.body.email)) {
res.status(400).send('Invalid email');
return;
}
// Email valid, create account...
})
Mailing List Subscribe
// Validate on mailing list signup
router.post('/subscribe', (req, res) => {
if (!emailValidator.validate(req.body.email)) {
res.status(400).send('Invalid email')
return;
}
// Passed, add to list...
})
Validating at data entry points prevents saving bad addresses that will just bounce later.
Verifying With the Mailgun API
You can also validate against Mailgun’s API to confirm active mailboxes:
const mailgun = require('mailgun-js');
const mg = mailgun({apiKey: API_KEY, domain: DOMAIN});
const isValid = await mg.validate('[email protected]');
if (isValid) {
// Passed, email is valid
} else {
// Failed validation
}
This actually connects to the recipient SMTP server to confirm the mailbox exists. Useful for scrubbing mailing lists.
Managing Hard vs. Soft Bounces
Once sending email, you’ll inevitably encounter some bounces. There are two main types:
Hard bounces occur when an email address is invalid or does not exist. These are permanent failures.
Soft bounces happen on temporary issues like a full inbox or inactive account. These may resolve later.
It’s important to handle these cases differently:
- Remove hard bounced addresses from your lists to avoid future issues.
- Retry soft bounces a few times before removing, as they may still be deliverable.
- Limit overall bounces to 5% or less to maintain good sender reputation.
By properly validating up front and managing bounces, you’ll avoid damaging your email deliverability through high failure rates.
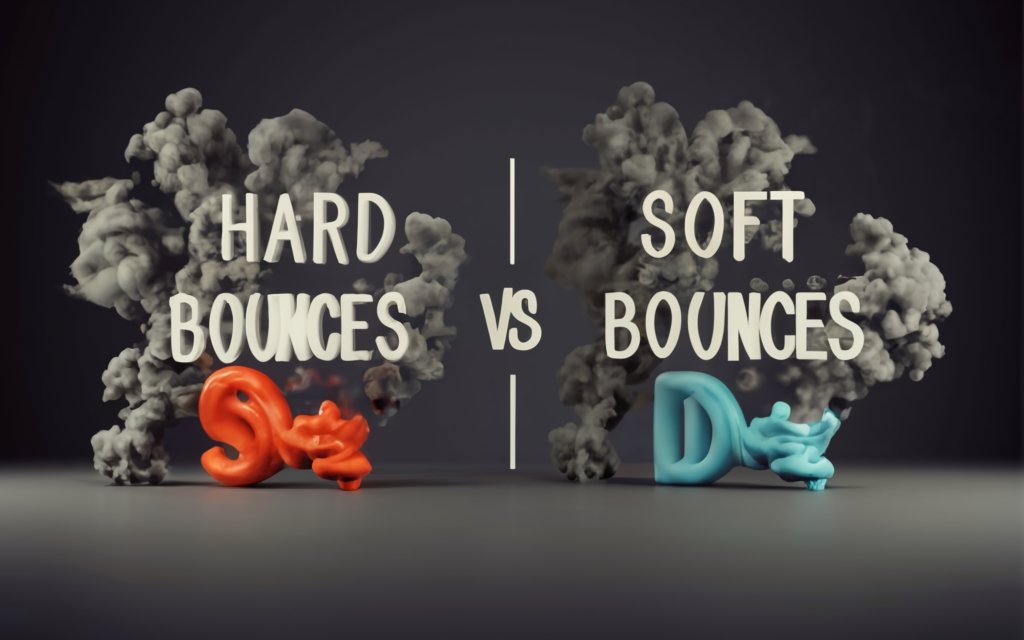
Using EmailJS for Opt-In Confirmation Workflows
One technique to keep address lists clean is requiring opt-in confirmation before fully subscribing someone.
You can implement confirmation workflows using the EmailJS npm package like:
// Send opt-in confirmation email
emailjs.send('template_id', template_params, YOUR_USER_ID)
This sends a pre-defined template to confirm the recipient’s address.
Once they click the link, you record them as a verified subscriber in your app.
Avoiding Spam Folders with Confirmation Emails
When sending out confirmation emails, there are some best practices to avoid the spam folder:
- Make sure FROM addresses match your domain, and are not no-reply style addresses.
- Send a personalized email with the visitor’s name in the body or subject line.
- Avoid using terms like ‘Free’, ‘Act Now’ or ‘Limited Time’ which can trigger spam filters.
- Consider adding the address to your email warmup list before sending the confirmation.
- Test with a personal email address to confirm deliverability before relying on confirmations.
Troubleshooting Failed or Missing Confirmations
Some issues to watch for with confirmation emails:
- Incorrect email address – Does the address match what the visitor provided?
- Spam filters – Check junk folders before resending.
- Request timeouts – Try increasing timeout limits in case of server latency.
- Request errors – Log and monitor failures like network errors.
- Incorrect template – Double check the right EmailJS template ID is being used.
With testing and monitoring, you can minimize lost or dropped confirmation emails.
Alternatives to EmailJS for Confirmation Templates
Besides EmailJS, some alternative Node.js services also provide template based transactional email:
- SendGrid templates – Drag and drop editor with dynamic template replacement.
- Mailgun templates – Inline or JSON based templates with templating syntax.
- MJML templates – Responsive email focused templating language.
- AWS SES – Templating features and workflow tools.
Each service provides their own twist like drag and drop editors, liquid syntax templates, batch sending tools, and more.
In Summary
Paying attention to email hygiene through verification and opt-in confirmation helps avoid deliverability issues from invalid addresses and excessive bounces. This protects your domain’s sender reputation, and ensures communication reliably reaches the intended recipients.
As your application’s email volumes grow, your basic SMTP server setup needs to scale as well. Choosing compatible email services, moving email off your app server, and leveraging services like SES helps optimize for reliable high volume sending.
Choosing an Email Service Matching Your Hosting
When evaluating third-party email delivery services like SendGrid or Mailgun, an important factor is integration with your hosting provider:
- Shared hosts – Options like SendinBlue, SMTP2GO, or Mailgun work well. AWS SES requires a dedicated IP.
- Cloud hosts like DigitalOcean – Leverage transactional email add-ons they offer or SMTP services like SendGrid.
- AWS EC2 – Use AWS SES with instance SMTP credentials for easy integration.
- Heroku – Add-on SendGrid or Mailgun for simple setup.
- Azure – Integrate Azure Email Service from the portal or SendGrid.
Matching your email service to the host streamlines configuration and avoids issues sending SMTP email through their infrastructure.
Moving Email Handling Off Your App Server
When starting out, it’s common to configure SMTP email directly within your Node.js app server. But as volumes increase, this can bog down performance.
Some options to offload email include:
- Dedicated SMTP VMs – Spin up separate virtual machines just for sending SMTP.
- Email services APIs – Call sending APIs from your app rather than running an SMTP server.
- Queueing – Queue in app, workers on separate servers process.
- Load balancers – Distribute SMTP connections across multiple servers.
Offloading helps isolate the resource demands of email from your main app. It also facilitates scaling capabilities.
Scaling Your SMTP Setup to Handle More Load
As your email needs grow, there are ways to scale your SMTP setup to handle greater loads:
- Horizontally scale – Add more servers behind a load balancer to distribute SMTP connections.
- Upgrade server sizes – Choose more powerful SMTP server instances with more resources.
- Enable throttling – Limit concurrent connections or recipients per domain to smooth traffic.
- Upgrade email plans – Raise email service package tiers for higher send limits.
- Optimize databases – Tune SMTP storage for speed if using a database like MySQL.
- Partition databases – Shard users across db clusters to distribute load.
- Enable caching – Cache email templates, recipients, and other data in memory.
With infrastructure tweaks like these, a basic SMTP setup can grow to handle considerable email loads.
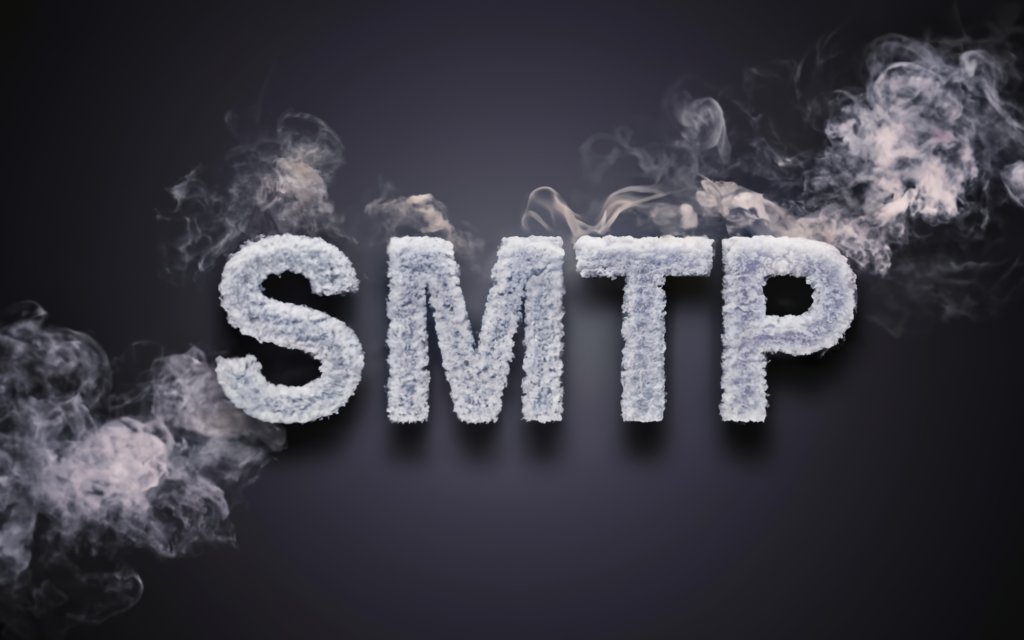
Monitoring Logs and Metrics
To stay ahead of issues at higher volumes, carefully monitor your SMTP activity:
- Error Tracking – Track failures and exceptions in services like Sentry.
- Email Logs – Inspect SMTP connection and delivery logs for anomalies.
- Throughput – Graph overall emails sent per minute/hour/day to spot increases.
- Rejected Recipients – Monitor address bounces and blocks by domain.
- Reputation Metrics – Watch blacklistings and spam complaints.
- Performance Stats – Graph SMTP server load, memory, response times to see bottlenecks.
Proper monitoring provides visibility into problems before they disrupt email flows.
Leveraging AWS SES for Bulk Email Delivery
For apps sending very high volumes, a service like Amazon SES can be a robust delivery option.
Some benefits of using SES include:
- Scalable cloud infrastructure – Built to handle enormous email volumes.
- SMTP integration – Generate SMTP credentials to use in Nodemailer.
- Cost effective – Very low cost per email sent.
- Automation – Rules and filters to manage bounces and reputation.
- Analytics – In-depth metrics on deliveries, spam reports, and engagements.
The key caveats are SES’s complex limits once past the initial sandbox stage. Throttling and queueing become necessary to avoid exceeding daily quotas. Still, the capabilities are unmatched for serious large scale email pipelines.
Avoid Blocks with Proper SES Warmup
When first using SES for high volume blasts, progress slowly to warm up IP reputations:
- Start with just a few hundred emails in the first week.
- Confirm emails are delivered reliably without issues.
- Gradually increase volume week over week.
- Mix different recipient domains as volumes grow.
- Keep growth consistent – don’t 10X send volume overnight.
Proper warmup minimizes the risk of hitting SES’s complex limits too soon. Take it slow.
Using SES SMTP Credentials in Nodemailer
To use SES in your Node.js apps, enable SMTP credentials:
// SES SMTP credentials
let transporter = nodemailer.createTransport({
host: 'email-smtp.us-east-1.amazonaws.com',
port: 587,
secure: false,
auth: {
user: 'AWS_ACCESS_KEY',
pass: 'AWS_SECRET_KEY'
}
});
These SMTP creds allow sending through SES just like any other service.
Managing SES Reputation and Sending Limits
As noted, AWS SES has intricate quotas and reputation systems:
- Sending limits based on your history and age of domain.
- Account level throttles on bounces and complaints.
- Complex cost implications once past sandbox.
Carefully manage:
- Warmup patterns to ramp volume gradually.
- Bounce and complaint rates to avoid account blocks.
- Reputation metrics across domains you send from.
- Cost by easing into higher tiers or consolidating volume.
Alternative Bulk Email Services
If SES seems too complex, other bulk sending options like:
- Mailgun – Great for early stage startups with flexible plans.
- SendGrid – Enterprise level deliverability with great support.
- SparkPost – Excellent analytics and adaptive delivery optimization.
While they can’t match SES scale, these providers are often an easier lift to get bulk sending capabilities up and running.
Final Takeaways
Configuring reliable delivery pipelines to handle peaks in traffic is crucial as your email needs grow. Plan your server setup, email service selection, and scaling approach accordingly.
With proactive performance monitoring and optimization, you can achieve great deliverability rates even at high volumes. Keep fine tuning based on data and your users will enjoy smooth email interactions with your application.
Sending generic blast emails leads to lackluster open and click rates. Personalizing content with tools like Mailgen helps craft unique emails programmatically.
Creating Stylish, Templated Emails with Mailgen
Mailgen is a Node.js package allowing you to generate stylish, responsive HTML emails using templating syntax:
const mailgen = require('mailgen');
// Configure email generator
let mailGenerator = new mailgen({
theme: 'default',
product: {
name: 'Mailgen',
link: 'https://mailgen.js/'
}
});
// Generate email with template
let email = mailGenerator.generate({
body: {
name: 'John Doe',
intro: 'Welcome to our app!',
action: {
instructions: 'Click here to get started',
button: {
color: '#22BC66',
text: 'Get Started'
}
}
}
});
// Send email
transporter.sendMail({
...
html: email
});
Mailgen handles creating good looking, responsive HTML emails using simple json-based templates.
Personalizing Email Content
To personalize content:
- Use merge tags like
{{name}}
in templates that pull data from JSON. - Swap out color schemes, fonts, and design based on customer.
- Customize images, headers, subject lines per user.
- Bake in dynamic data like account info, order details etc.
- Segment users for different email flows or special offers.
The more targeted the content, the higher engagement and click through rates will be.
Avoiding Spam Triggers
When designing and sending bulk transactional email:
- Avoid spammy keywords like “Act Now!” in subjects.
- Use natural language in sentences – no ALL CAPS.
- Personalize subject lines and content rather than blasting clones.
- Make sure unsubscribe links work and are present.
- Send from domains users recognize and expect mail from.
- Consider warmup techniques to build sender reputation slowly.
Following best practices improves deliverability and avoid spam folderings.
Testing and Troubleshooting Templated Email
When sending dynamic email:
- Test by sending to yourself first before mass sending.
- Render the final HTML and preview how it will look.
- Check spam folders to identify any filter triggers.
- Trace merge tag problems back to incorrect JSON data.
- Adjust template styling that looks broken or inconsistent.
- Log and monitor failures to improve template robustness.
Testing allows catching mistakes and optimizing the templates for best results.
Other Templating Tools Like MJML
Besides Mailgen, some alternative HTML email templating tools include:
- MJML – Responsive email focused framework with React components.
- Pug – Templating engine that can generate HTML.
- Mustache – Logic-less templates for HTML email bodies.
- Marko – Templating and UI components optimized for HTML email.
- EmailJS – Node package for email templating and sending.
Each provides different sets of layouts, components and dynamic data injection models.
Balancing Personalization With Batch Sending
The tension with templated, personalized email is automating generation versus mass blasting clone-like messages.
Here are some best practices for balancing both:
- Build 1 standard template per email type like “Welcome”, “Reset Password” etc.
- Pull in data dynamically like customer name, account details etc.
- Swap color schemes or images to match brands being emailed.
- A/B test different subject lines, call-to-action phrasing.
- Add some randomness – not every email needs 6 dynamic fields.
The goal is to avoid repetitive template spam while still allowing easy batch sending.
A/B Testing Email Content
To discover the highest performing email content, A/B test factors like:
- Subject line phrasing – test urgency, length, emojis etc.
- Different calls-to-action – “Get Started” vs “Begin Trial” vs “Sign Up”.
- Email templates and designs – colors, images, fonts.
- Content sections – test including certain paragraphs.
- Offers or discounts – savings amount, expiration dates.
Then send half the list version A, the other half version B. See which generates more opens, clicks, and conversions to further optimize.
Using Tools to Dynamically Generate Email
To inject dynamic data into emails, use templating tools like:
- Node-Email-Templates – Inline template replacement.
- MJML – React based responsive email components.
- Pug – Mix static and dynamic content.
- Mailgen – JSON and handlebars template injection.
- MailMerge – Personalize bulk Word documents as email bodies.
Choose the syntax fitting your use – inline, JSX components, handlebars etc.
Ensure Uniqueness in Each Email
Avoid sending generated emails that look cloned or robotic:
- Personalize emails even if just using first name.
- Vary offers, discounts, content sections.
- Randomly select color schemes, hero images per email.
- Craft subject lines relevant to each customer.
- Follow up individually on customer issues mentioned.
- Thank new customers personally for signing up.
With just a bit of variability and personalization, you can automate email generation while still providing a human touch.
In Summary
Well designed email templates injected with dynamic content optimize engagement while allowing efficient batch sending. Use tools like Mailgen and MJML to create great looking, responsive templates that avoid spam triggers. Work in personalization with customer data to boost open and click through rates. With smart templating and automation, you can achieve highly targeted, yet scalable email campaigns.
Here are the key takeaways from this comprehensive guide on troubleshooting Gmail delivery issues:
- Gmail’s queuing system prioritizes and throttles message sending behind the scenes. Emails may be briefly delayed for reasons like priority, spam analysis or traffic shaping.
- Nodemailer provides a straightforward way to send email from Node.js apps. However, proper configuration and testing is crucial to avoid errors. Watch for ECONNREFUSED connection issues.
- Services like Mailgun simplify delivery at scale, but have usage limits that can trigger blocks if exceeded. Implement throttling and sender warmup best practices.
- Validating email addresses upfront prevents damaging bounces and reputation loss later. Manage hard vs soft bounces accordingly.
- Move email handling off your main app instances. Horizontally scale SMTP setups to handle more load by adding dedicated servers.
- For high volume needs, leverage services like AWS SES. But be mindful of complexity in managing reputation, quotas and costs.
- Tools like Mailgen allow creating stylish, engaging transactional emails from templates. Personalize content while avoiding spammy email blasts.
- Monitor key metrics like spam complaints, blocks, bounces and latency. Optimize configurations continually based on data.
Delivering email reliably at scale requires diligent performance tuning, capacity planning and error handling. Follow these best practices and your Node.js apps will send email smoothly without deliverability headaches.
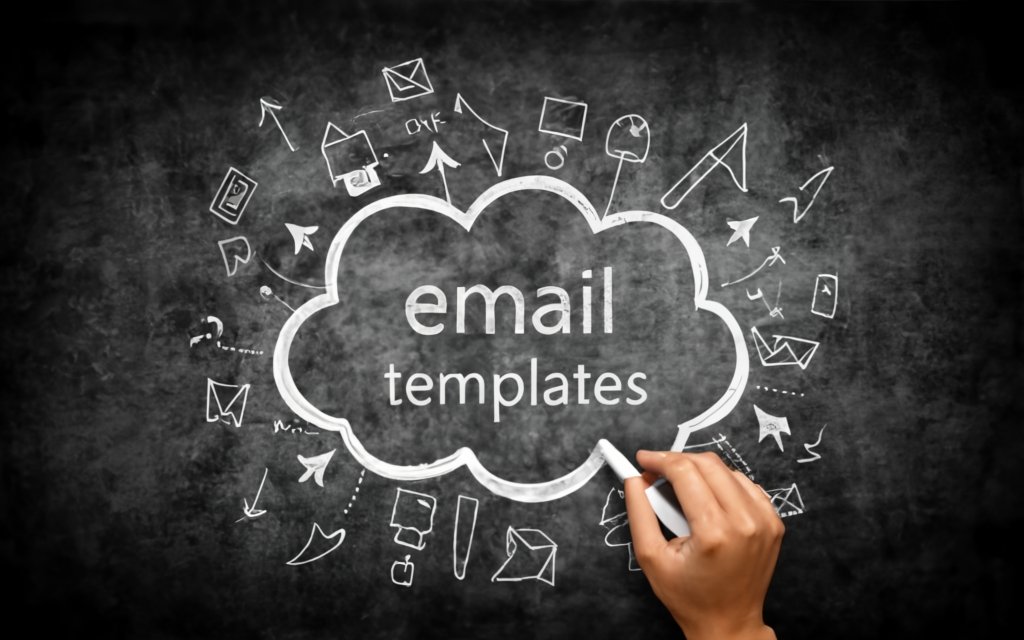
Here are some frequently asked questions about troubleshooting Gmail delivery issues:
Why are my Gmail messages getting stuck in the queue?
Gmail may intentionally delay sending emails for reasons like spam analysis, traffic shaping, or priority. Queue delays are typically brief but can stretch longer during peak periods. Large attachments, bulk recipients, and new addresses are common factors for queued messages.
I’m getting ECONNREFUSED errors in Nodemailer. How do I fix this?
Connection refused errors mean Nodemailer cannot establish a connection to the SMTP server. Double check your hostname, port, and SSL settings match your email service. Also try testing basic SMTP connectivity with Telnet. Switching SMTP services like using Mailgun can help determine if the problem is server specific.
My Mailgun account was disabled – what should I do?
If you exceed Mailgun’s usage quotas too quickly such as sending 5k emails immediately, they will disable accounts. Allow your account to reset overnight or contact support. For the future, implement throttling, queueing, and proper warmup to ramp up gradually.
Why do I need to validate emails before sending?
Validating email addresses prevents send failures and bounces which harm your sender reputation. Check format validity with libraries like validator. You can also validate existence by connecting to SMTP servers with the mailgun API.
How can I optimize my app servers for heavy email sending?
Consider moving email handling to separate servers to reduce load on your app instances. Horizontally scale by adding more SMTP servers behind a load balancer. Monitor metrics like errors, latency and throughput to catch bottlenecks.
When should I use a service like AWS SES for sending high volume email?
SES provides nearly unparalleled scale, but has a complex reputation and quota system. It can be overkill for many needs. Start with a service like Mailgun or SendGrid unless you anticipate spikes above 100k emails per day. Gradually warm up any service to build reputation.
Why aren’t my opt-in confirmation emails getting delivered?
Check for typos in the recipient address. Also try verifying the email reaches your own inbox vs spam folder before mass sending. Increase timeout limits in case of server latency issues. Log errors for troubleshooting.