Tired of wrestling with cryptic bounce messages and unsubscribes? VERP provides a clever way to optimize email deliverability through address encoding. Learn how it works and if it’s right for your program.
What is VERP (Variable Envelope Return Path)?
Dealing with email bounces and undeliverable addresses is the bane of most email marketers’ existence. Analyzing bounce messages to extract the failed addresses, then piecing together which records bounced can be tedious and time-consuming. But what if there was a better way? Enter VERP – Variable Envelope Return Path.
VERP is an ingenious email technique that allows senders to automatically detect bounced addresses and clean their lists without needing to manually analyze bounce messages. Let’s break down how it works and its key benefits for deliverability.
How VERP Encodes Recipient Addresses
The crux of VERP lies in encoding information within the return path, also known as the envelope sender or “MAIL FROM” address used in the SMTP transaction. This return path is hidden from recipients, but visible to mail servers.
With VERP, the sender dynamically generates a unique return path for each recipient by encoding their email address within it.
For example, when sending an email to [email protected], the return path may be:
[email protected]
If Katherine’s email is [email protected], her return path would be:
[email protected]
So each recipient gets a customized return path containing their address.
Automated Bounce and Complaint Handling
When an ISP tries to deliver to an invalid recipient address, the bounce message goes back to the associated return path.
Since this encoded return path contains the original recipient’s email, the sender immediately knows which address bounced without needing to parse the bounce message content.
The same applies for complaint feedback loops. If [email protected] clicks “report spam”, the complaint notification goes to the return path containing katherine’s encoded address.
This automation makes bounce and list hygiene management much simpler at scale.
Optimizing Deliverability with Major Providers
VERP facilitates deliverability by enabling better feedback loops with major inbox providers.
Gmail, Outlook and others leverage these encoded return paths to identify problematic senders abusing their systems.
Senders using VERP show they actively monitor bounces and complaints, gaining trust with providers. This can improve inbox placement and avoid negative impacts from future misbehaving recipients.
Yahoo Mail’s Feedback Loop system directly relies on VERP-encoded return paths to report complaints at scale.
Use Cases and Applications
VERP shines for high-volume email programs like:
- Newsletters and promotional campaigns
- Transactional notifications like order confirmations
- Bulk email services blasting to lists
It streamlines list hygiene for constantly changing recipient cohorts. Sending one-off emails doesn’t gain as much advantage.
Facilitating unsubscribe and complaint handling is also a prime use case. Encoding addresses allows immediate removal of recipients requesting to opt-out.
Further, VERP enables better inbox placement with whitelist providers like Return Path’s Certification program. These programs prioritize senders actively monitoring reputation via VERP.
Now that we’ve covered the fundamentals, let’s look at some implementation details.
Technical Overview
On a technical level, VERP requires dynamically generating a unique return path and supplying it during the SMTP transaction.
Unique Return Paths
Some best practices for structuring VERP return paths:
- Encode recipient address at the end after an “=” sign
- Prefix with a static label like “bounce-” to identify VERP bounces
- Keep the domain consistent, often using a general mailbox
So [email protected]
works well.
Specifying During SMTP
The key is specifying this encoded return path as the “MAIL FROM” when handshaking with the receiving SMTP server.
Standard libraries like Python’s smtplib don’t directly support overriding the return path. So it requires a custom SMTP client or script.
Libraries like Mailgun’s Pygments fully support supplying VERP return paths during SMTP.
Handling Encoded Bounces
Once VERP is implemented, delivered bounce messages will have encoded return addresses.
These can be automatically parsed to extract the original failing recipient address without analyzing the bounce content itself.
The Bottom Line
VERP takes bounce management from an opaque art to a transparent science. Encoding addresses removes the hassle of deciphering confusing bounce messages or waiting for complaints.
The outcome? Cleaner lists, improved deliverability, and more time spent crafting great email content vs fighting fires.
While it involves some upfront effort to implement, VERP pays long-term dividends for high-volume email programs. The deliverability gains often justify the costs.
In the next sections, we’ll cover some best practices for usage, pricing considerations, and the future of this address encoding technique.
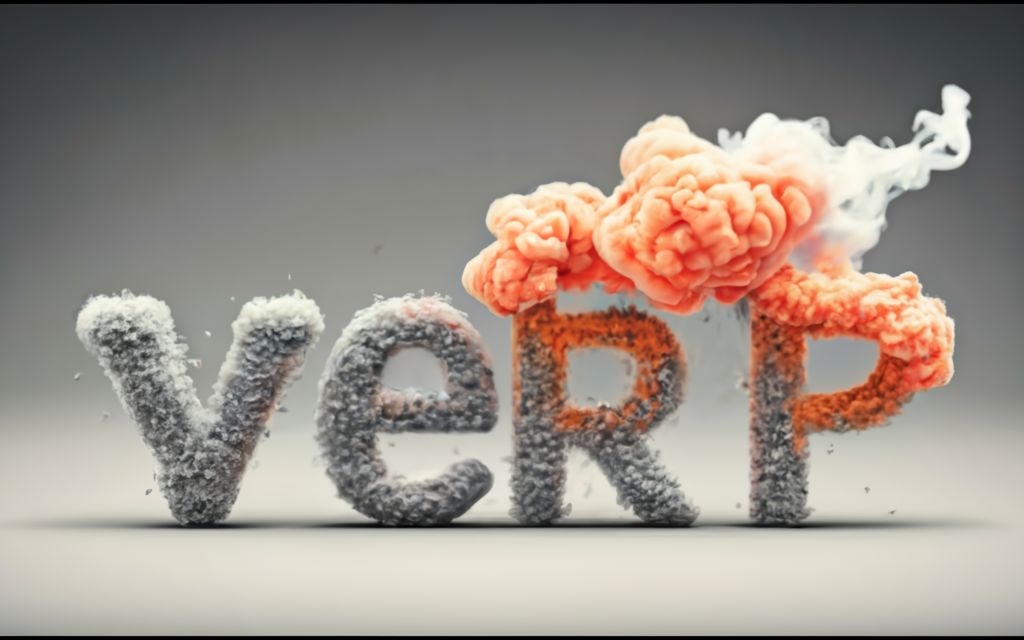
VERP Implementation and Technical Details
Now that we’ve covered the high-level value of VERP, let’s get into the nitty-gritty of how it works under the hood. We’ll look at:
- How recipient addresses get encoded into return paths
- Generating unique MAIL FROM addresses programmatically
- Handling bounces and unsubscribes from encoded data
- The role of SMTP protocol in VERP messaging
- Sending VERP emails from code using various languages and tools
Understanding these technical elements will help you evaluate if implementing VERP is feasible for your email program.
Encoding Recipient Addresses in the Return Path
The first step is constructing a return path (envelope sender) that encodes each recipient’s email address.
There are two main strategies here:
Encoding the Full Address
You can encode the entire recipient address like:
[email protected]@mydomain.com
Pros:
- Uniquely identifies each recipient
Cons:
- Return path length may hit SMTP max limit of 255 chars
- Encodes potentially private info in path
Encoding Just the Domain
A shorter approach is encoding only the domain:
[email protected]
Pros:
- Shorter return path length
- Omits private info from encoding
Cons:
- Can’t distinguish recipients at same domain
Given length limits, encoding just the domain is generally preferred. You can still identify the full address later when parsing bounces.
Best Practices
Some other tips for constructing VERP return paths:
- Prefix with a label like “bounce-” to easily identify VERP bounces
- Keep a consistent sender domain for your MAIL FROM paths
- Use a general mailbox that accepts all VERP bounces
With encoding schemes decided, let’s look at generating unique paths.
Programmatically Generating Unique Return Paths
For each outbound email, you’ll need to dynamically construct a return path with an encoded recipient address.
Pseudocode for this logic may look like:
recipient = "[email protected]"
// Extract domain
domain = recipient.split("@")[1]
// Create encoded return path
returnPath = "bounce-" + domain + "@mycompany.com"
You’ll want to incorporate this generation into your email sending loop. Most modern ESPs have libraries or plugins that handle encoding under the hood.
Supplying VERP Return Paths via SMTP
Next we need to supply this encoded return path as the MAIL FROM when transmitting the message.
SMTP Protocol Basics
When sending email via SMTP, the client and server handshake follows this process:
- Client initiates session
- Client supplies sender address with MAIL FROM
- Client supplies recipient address with RCPT TO
- Server confirms accepted addresses
- Client sends message content
The MAIL FROM is where we provide our VERP encoded return path.
Limitations of Standard SMTP Libraries
Most standard SMTP libraries like Python’s smtplib don’t let you override the MAIL FROM.
They use the message’s From address both for the SMTP envelope sender and as the visible email header From.
Custom SMTP Scripts
To fully control the envelope sender, we need a custom SMTP client that supports passing the return path as a separate parameter.
Python’s smtplib does have an undocumented mail_options
arg that can override the envelope sender on some servers.
SMTP Client Libraries with VERP Support
Many ESPs provide custom libraries with VERP support like Mailgun’s Pygments and Amazon SES’s Boto3.
These libraries handle constructing encoded return paths and supplying them via SMTP.
Parsing Encoded Bounce Addresses
Once VERP is implemented, bounce messages will come back to your encoded return paths.
These can be programmatically parsed to extract the original recipient address that bounced.
Pseudocode:
// Extract encoded address
returnPath = "[email protected]"
// Split on '@' and '-'
domain = returnPath.split("@")[1].split("-")[1]
// Reconstruct address
originalRecipient = "anything@" + domain
The same parsing logic works for identifying unsubscribe requests from complaint feedback loops.
This automation removes the need to manually analyze raw bounce messages.
The Path Forward
The technical side of VERP may seem daunting, but modern tools continue simplifying implementation.
For many ESPs, enabling VERP is now a checkbox rather than a coding project.
The results are worth the effort: cleaner lists, smoother unsubscribes, and deliverability gains that improve engagement.
In the next section, we’ll explore pricing models for VERP services and how to determine potential ROI.
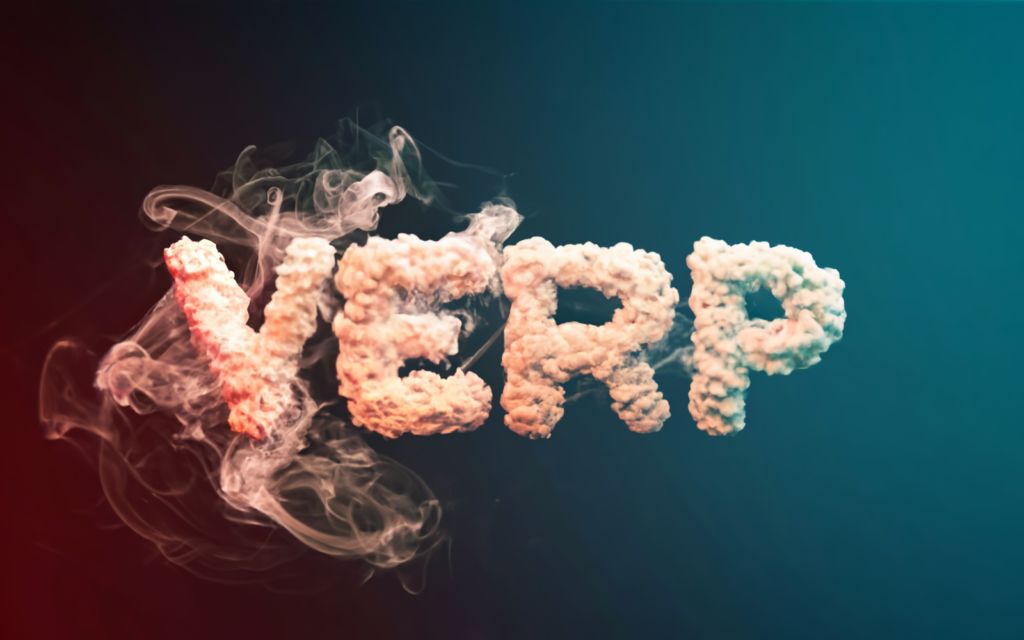
Pricing and Cost Considerations for VERP Services
One key question around VERP is what the service costs, and whether the deliverability benefits outweigh the added complexity. In this section, we’ll explore:
- Pricing models from top VERP providers like Return Path
- Cost factors related to email volume and features
- Calculating potential return on investment (ROI)
- Qualitative benefits beyond hard financial metrics
With this context, you can determine if VERP makes economic sense for your email program.
Return Path Certification Pricing
Return Path offers the most widely used VERP certification service. Their pricing tiers are:
- Elements – $20/mo base fee
- Elements Plus – $525/mo base fee
- Professional – Custom quote based on usage
- Enterprise – Custom quote based on usage
The main factors that influence cost are:
Email Volume
More emails means more encoded return paths and bounces to handle. High-volume Pro and Enterprise plans get custom quotes.
Feature Sets
Higher tiers include added capabilities like compliance reporting and predictive inbox placement. Advanced features increase costs.
Support and Integrations
Extras like dedicated account management and ESP integrations add to the price tag.
So costs range from $240/year for basic VERP certification up to five-figure contracts for enterprise-grade implementations.
Self-Implementation and Other Providers
Rather than use Return Path, some companies choose to own their VERP infrastructure.
Major ESPs like Mailgun, SendGrid, and Mailchimp provide programmatic APIs for encoding return paths. Building on top of these can be more affordable for high email volumes.
Similarly, leveraging cloud messaging services like Amazon SES to enable VERP may have lower costs than Return Path’s tiers.
The trade-off is losing the potential certification benefits and having to build and manage everything in-house.
Evaluating ROI of VERP
To determine if the price is justified, consider both the hard cost savings and qualitative benefits.
Calculating Deliverability Improvements
What’s the value of improving your inbox placement rate by 2%, 5% or 10%? More inboxes means more engagement.
With that lift quantified, tally the decreased costs for re-engaging bounced users through other channels. This estimates deliverability ROI.
Valuing Subscriber Retention
Unsubscribe handling also retains more net subscribers over time. Estimate the lifetime value of those saved subscribers no longer churning due to friction.
Qualitative and Operational Gains
Simpler bounce management saves hours parsing complicated messages. And your team spends less time troubleshooting deliverability fires.
Evaluate both the hard and soft factors to determine if VERP delivers a positive ROI. For most large email programs, it does given today’s congested inboxes.
Finding the Right Fit
VERP shines for high volume emailers, where small deliverability gains have big bottom line impact.
For lower volumes, assess if DIY implementation on top of an ESP like Mailgun makes sense. This can provide VERP power at an affordable price.
The road to inbox success involves balancing many factors. But for most serious email marketers, VERP’s benefits are well worth the investment.
Next we’ll switch gears to cover some best practices and limitations to be aware of with VERP campaigns.
Best Practices and Limitations of VERP
VERP is a powerful technique, but also has some limitations and nuances to consider. In this section we’ll explore:
- Usage best practices to maximize deliverability benefits
- Drawbacks like single recipient messaging requirements
- Complementary approaches to further enhance inbox placement
- How to evaluate if VERP aligns with your email program’s needs
Understanding the pros and cons will help you make an informed decision about VERP adoption.
Recommended Usage Guidance
While VERP works “out of the box”, following some best practices maximizes effectiveness:
Keep Individual Messages Under 10 Recipients
Major ISPs like Gmail prioritize small recipient batches. For VERP blasts, group emails into smaller segments under 10 recipients each.
Have Realistic Content Variability
Too much variability between recipients signals potential spam or unsafe lists. But some personalization and merges help demonstrate engaged subscribers.
Send at Moderate Cadences
Frequent messaging and batch sends can trigger abuse filters. Blend VERP mailings with more steady cadences.
Double Opt-In Subscriptions
Requiring explicit confirmation when subscribers join demonstrates engaged audiences. This boosts reputation with inbox providers.
Monitor and Optimize Engagement
Even with VERP, actively respond to feedback on failed messages and engagement drops. Continuously tune content and segmentation.
Following these guidelines will maximize VERP’s deliverability advantages.
Potential Drawbacks and Limitations
VERP also comes with some inherent limitations to factor in:
Recipients Must Each Get Unique SMTP Connection
Because a unique MAIL FROM is required per recipient, SMTP connections can’t be reused across recipients in the same JOB. Some use cases like email blasts may require more connections.
Supplementary Techniques Still Needed
VERP complements other deliverability tactics like seed lists, inbox monitoring, and sender reputation tracking. It doesn’t eliminate the need for these.
Adds Complexity to Deployments
There’s overhead to integrating VERP properly at the infrastructure and application layers. For simpler programs, this may not justify benefits.
Understanding these constraints allows aligning expectations and mitigating issues early on.
Alternatives to Evaluate
For some use cases, supplementary or alternative approaches may work better than VERP:
Standard Bounce Processing
Basic bounce handling without VERP still gives list hygiene benefits, though requiring manual analysis.
Limited Seed Lists
Small seed lists to benchmark inbox placement help identify deliverability drops before sending full blasts.
Signal Monitoring
Email authentication, links, and tracking signals help gauge reputation without VERP encoding specifically.
Inbox Providers’ Feedback Loops
Services like Gmail Postmaster Tools share aggregated complaint and spam reporting data.
These options have lower overhead than full VERP implementation. But they miss out on some personalized optimization benefits.
Assessing VERP Fit
Given the pros, cons and alternatives – how do you know if VERP is right for your program?
Key factors to weigh:
Current Deliverability and Engagement
If inbox rates are consistently high and engagement solid, VERP gains may be marginal. Focus on sustaining performance first.
Volume and Importance of Email Channel
For lower volume programs where email isn’t make-or-break, VERP’s overhead likely isn’t justified.
Availability of Resources and Expertise
For resource-constrained teams without deliverability expertise, VERP may ultimately degrade performance if improperly implemented.
Balancing these dynamics will guide smart decisions on investing in VERP.
While not a silver bullet, VERP remains a valuable tool for elevating sophisticated email programs.
In the final section, we’ll look at what’s ahead – the future of VERP technology and practices.
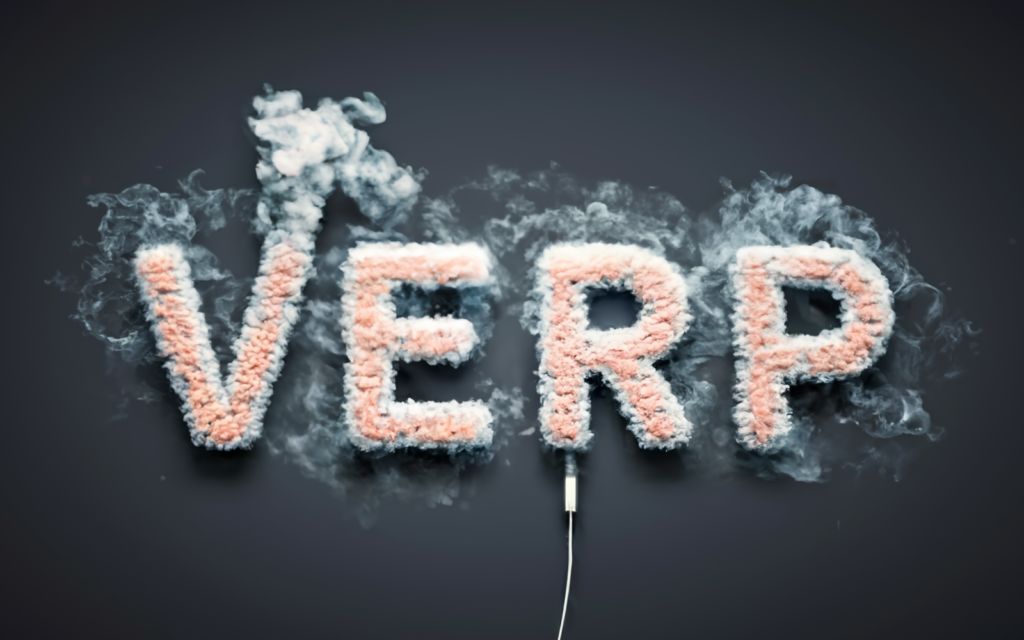
The Future of VERP in Email Deliverability
VERP has cemented itself as an email best practice for major senders. But where does the technology go from here? What emerging trends and techniques could augment or potentially displace VERP’s role?
In this final section, we’ll look at:
- How inbox providers are leveraging VERP data
- The potential impact of new standards like ARC
- What the future could hold for VERP and address encoding
Understanding these dynamics will help you stay ahead of the curve and maximize long-term deliverability.
Expanding Use of Encoded Data by Receivers
Major webmail providers are finding new ways to leverage VERP’s encoded information to filter messages:
Scoring Recency of Encoded Addresses
Google and others factor how recently an encoded address was active to detect potentially unsafe lists. Fresher data looks more trustworthy.
Parsing Encoded Metadata
Information beyond the recipient address can be encoded and extracted to enhance context and filtering decisions.
Correlating Encoded Info Across Senders
Collating VERP data from many senders helps identify busy mailboxes and globbal spam operations.
As adoption spreads, expect receivers to further optimize VERP’s usage and value.
Emergence of ARC Standard
ARC or Authenticated Received Chain offers an emerging standard that overlaps with elements of VERP.
It focuses on cryptographically signing key components like:
- Original sender
- Receiver
- Return path
This allows validating the authenticity of routing info as messages get transferred between hops.
If adopted at scale, standards like ARC could complement or partially displace VERP in the future. Though universal adoption remains early.
Ongoing Deliverability Innovation
The cat-and-mouse game of deliverability shows no signs of slowing down.
As receivers evolve new filtering techniques, senders reply with innovations in encoding, authentication, and behavioral modeling.
We can expect continued advancement around:
- Optimized and expanded info encoding
- Increased usage of cryptography and signatures
- Behavioral modeling of sender patterns
Likely no one silver bullet displaced VERP entirely. But its scope and role may shift as part of broader deliverability infrastructure modernization.
The Path Ahead
Email is far from a solved problem. Recipient habits and expectations continue rising even as volumes swell.
Deliverability gains require constant iteration, not one-time fixes. VERP represents an important tool, but not the end of the road.
By staying up-to-date on techniques like encoding and authentication, senders can sustain trusted reputations and maximize reach.
The specifics will change over time, but the fundamentals remain: Build great subscriber experiences, monitor and optimize engagement, and keep innovating new solutions.
With these principles, your program is poised for inbox success both today and in the future.
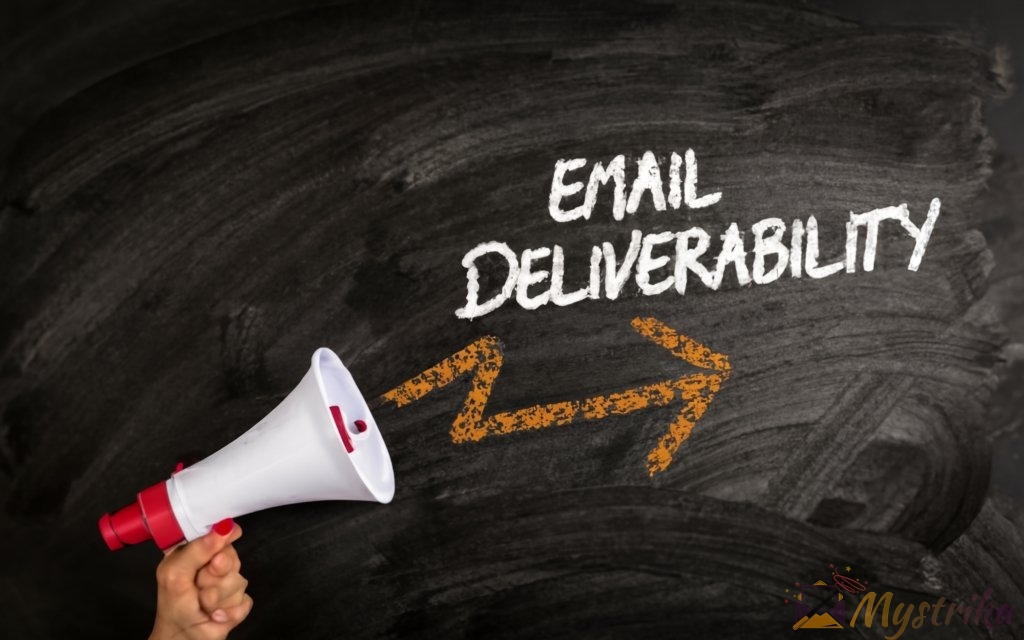
Key Takeaways
VERP provides a powerful method for improving deliverability, but has nuances to consider before implementation. Some core lessons:
- Encoding recipient addresses in return paths automates bounce and complaint processing. This streamlines list hygiene.
- Major inbox providers leverage VERP data to identify quality senders. This can improve inbox placement.
- Adding VERP requires generating unique SMTP MAIL FROM addresses and handling encoded bounces programmatically.
- For high volume mailers, deliverability gains often offset VERP costs of $20-$500+ per month from vendors.
- Alternatives like seed lists and standard bounce processing offer lower overhead depending on needs.
- Usage best practices around segmentation, engagement monitoring, and moderate send cadence optimize results.
- No silver bullet exists – VERP complements emerging authentication approaches and ongoing optimization.
- Regularly evaluate VERP costs and benefits as program needs evolve.
Email challenges continue growing. But techniques like VERP provide the building blocks for addressing them. Combined with audience understanding and great content, deliverability and engagement goals remain in reach.
Frequently Asked Questions
What are the major benefits of VERP?
The main benefits are automated bounce and complaint handling to keep lists clean, increased deliverability from whitelisting, and reduced overhead analyzing raw bounce messages.
What extra work is required to implement VERP?
Key tasks include generating unique return paths per recipient, modifying SMTP delivery to use encoded paths, and parsing bounced addresses from VERP strings.
Does VERP work for any size email program?
VERP provides the most value for larger email volumes above several thousand messages per month. The overhead likely isn’t justified for very small programs.
Can I use my existing ESP to enable VERP?
Many major ESPs like Mailgun, SendGrid, and Mailchimp provide APIs and plugins to utilize VERP capabilities on their platforms.
Is VERP compatible with other deliverability techniques?
Absolutely. VERP complements other tactics like seed lists, inbox monitoring,spam checks, and sender authentication.
What happens if I enable VERP improperly?
If implemented incorrectly, VERP risks damaging deliverability through authentication failures or triggering spam filters. Have technical experts oversee integration.
What alternatives should I consider beyond VERP?
For lower complexity, options like standard bounce processing, limited seed lists, signal monitoring, and feedback loops from receivers can help.
How does VERP work if recipients use forwarding or aliases?
Most ESPs will rewrite the envelope sender with any forwarding info from preprocessing. So the VERP encoding remains intact end-to-end.
Can I selectively enable VERP for some recipients and not others?
Segmenting is possible but adds further complexity. Generally it’s best to be consistent in approach across your list for simplicity.
What emerging technologies could impact VERP in the future?
Standards like ARC for cryptographic signing may shoulder some of VERP’s authentication duties down the road. But adoption is early.