Have you ever struggled with handling emails, notifications, and alerts in Django? Do invalid email addresses, unreliabled deliverability, or poor inboxing rates give you nightmares? This comprehensive guide will turn you into a Django messaging master – from sending the perfect transactional emails to building scalable notification systems and everything in between! We’ll explore Django’s built-in messaging framework, email best practices, integration with external providers, scaling high-volume delivery, and tons more. By the end, you’ll have the confidence to tackle any messaging need in Django with ease. Let’s get started!
Overview of Django’s Built-In Messaging Framework
Django provides a robust messaging framework out of the box to display timely notifications, alerts, and other messages to users. The framework is flexible enough to support messages for both authenticated and anonymous users.
Enabling Messages in Django
Integrating messaging in a Django project is straightforward. Here are the steps:
- Add
'django.contrib.messages'
to yourINSTALLED_APPS
setting. - The middleware classes
SessionMiddleware
andMessageMiddleware
should be present inMIDDLEWARE
. - Include
'django.contrib.messages.context_processors.messages'
in thecontext_processors
option of theDjangoTemplates
backend defined inTEMPLATES
.
That’s it! The messages framework will be ready to use.
Configuring Message Storage Backends
By default, messages are stored in the session. But the storage backend can be customized as per the use case. The built-in storage options are:
SessionStorage
– Stores messages in the request’s session. Requires thecontrib.sessions
app.CookieStorage
– Stores message data in signed cookies. Falls back to session storage if the cookie data exceeds 2048 bytes.FallbackStorage
– Uses cookies if possible, otherwise falls back to session storage.
The default storage class is FallbackStorage
. To use a different backend, set the MESSAGE_STORAGE
setting:
MESSAGE_STORAGE = 'django.contrib.messages.storage.cookie.CookieStorage'
Adding and Displaying Messages
Adding messages is simple using the messages
framework:
from django.contrib import messages
messages.debug(request, '%s SQL statements were executed' % count)
messages.info(request, 'Your account expires in 3 days')
messages.success(request, 'Profile updated')
messages.warning(request, 'Invalid credentials')
messages.error(request, 'Document deleted')
To display messages in a template, iterate through them:
{% if messages %}
<ul class="messages">
{% for message in messages %}
<li{% if message.tags %} class="{{ message.tags }}"{% endif %}>
{{ message }}
</li>
{% endfor %}
</ul>
{% endif %}
The message content and level tags are conveniently available in the template.
Creating Custom Message Levels
The built-in message levels (debug, info, success, warning, error) can be extended by creating custom levels.
For example:
CRITICAL = 50
def my_view(request):
messages.add_message(request, CRITICAL, 'A critical error occurred.')
Just don’t override the numeric values of existing levels.
The new level name and tag can be configured via the MESSAGE_TAGS
setting.
Setting Message Expiration
By default, messages are cleared when retrieved and displayed. To persist them across requests, set the storage instance to false after iteration:
“`python
storage = messages.get_messages(request)
for message in storage:
# …
storage.used = False
This prevents the messages from being marked as read.
The messages framework is quite handy for short-term situational messages. With some smart configuration, it can be tailored to messaging needs of any project.
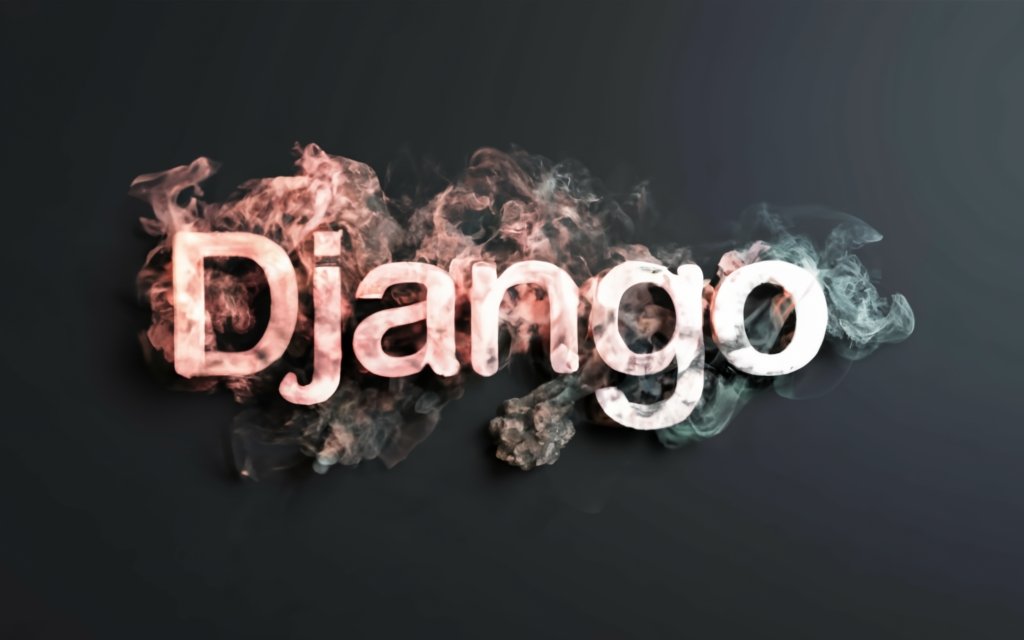
Sending Emails from Django
Django provides a simple API for sending email right out of the box. With just a few lines of configuration and code, your application can start sending transactional, bulk, and templated emails.
Setting up SMTP Configuration
To send emails, Django needs to be configured with SMTP server details. The mail server settings are:
EMAIL_HOST - SMTP server domain name e.g. smtp.example.com
EMAIL_PORT
- SMTP port number (default is 25)EMAIL_HOST_USER
- Username for authenticationEMAIL_HOST_PASSWORD
- Password for authenticationEMAIL_USE_TLS
- Enables TLS encryption (True or False)
For Gmail, the settings would be:
EMAIL_HOST = 'smtp.gmail.com'
EMAIL_PORT = 587
EMAIL_HOST_USER = '[email protected]'
EMAIL_HOST_PASSWORD = 'your_gmail_password'
EMAIL_USE_TLS = True
Make sure two-factor authentication is turned off for your Gmail account.
Sending Basic Emails
With SMTP configured, sending a simple email is straightforward:
from django.core.mail import send_mail
send_mail(
'Subject',
'Body goes here',
'[email protected]',
['[email protected]'],
fail_silently=False,
)
The send_mail
function takes subject, message, from email, recipient list, and fail silently flag.
Adding Attachments
To send email attachments, use the EmailMessage
class instead:
from django.core.mail import EmailMessage
email = EmailMessage(
'Subject',
'Body',
'[email protected]',
['[email protected]'],
)
email.attach_file('path/to/file.pdf')
email.send()
Call attach_file()
for each file to attach before sending the email.
Using EmailMessage and EmailMultiAlternatives
For more advanced email features, the EmailMessage
and EmailMultiAlternatives
classes are handy:
from django.core.mail import EmailMultiAlternatives
email = EmailMultiAlternatives(
'Subject',
'Text body',
'[email protected]',
['[email protected]']
)
email.attach_alternative('<p>HTML body</p>', 'text/html')
email.send()
This sends a multipart email with both text and HTML versions.
Leveraging Templated Email Content
For templated emails, pass the template name and context to render_to_string() and use the rendered output as the email body:
from django.template.loader import render_to_string
email_body = render_to_string('email_template.txt', {
'name': 'John',
})
send_mail(
'Subject',
email_body,
'[email protected]',
['[email protected]'],
)
This approach avoids repeating fixed email content.
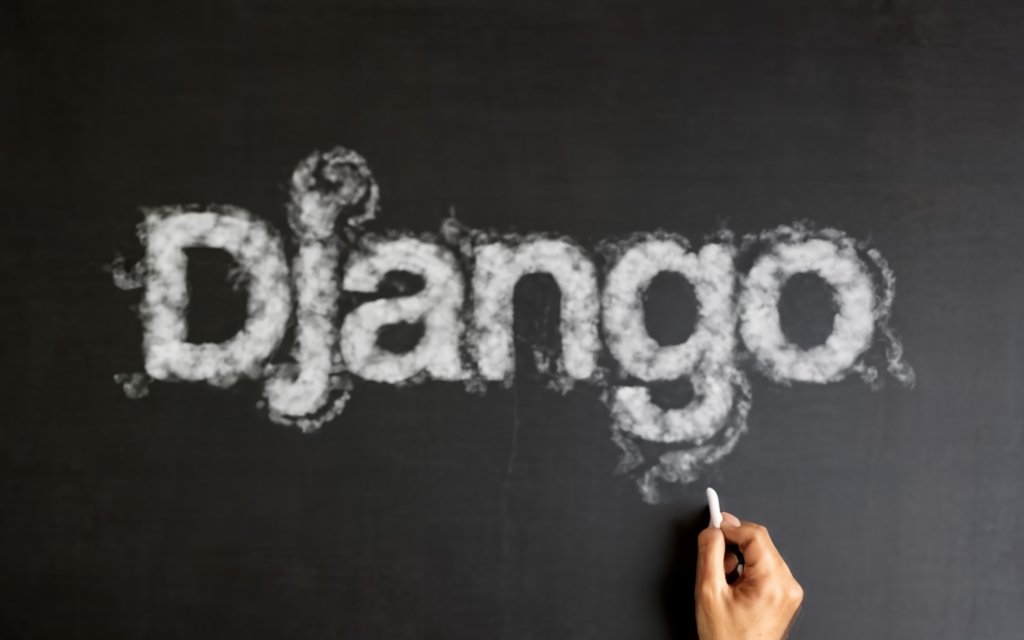
Email Deliverability Best Practices
Getting your application emails reliably delivered into the recipient's inbox is vital. Here are some tips and best practices to improve email deliverability.
Warming Up New Email Addresses
When first using a new sending domain or email address, don't immediately blast out mass emails. First, warm up the IP and domain reputation.
Warmup strategies:
- Send a small volume of 5-10 emails per day to verify addresses.
- Use services like Mailgun's warmup feature to improve deliverability.
- Rotate warmed-up IPs to maintain good reputation.
This builds a legitimate sending profile and minimizes the risk of spam filtering.
Avoiding Blacklists
Getting blacklisted by ISPs or spam filters leads to detrimental delivery issues. Some ways to avoid blacklists:
- Check blacklists regularly and remove any blocked IPs.
- Ensure proper SPF and DKIM setup to authenticate your domain.
- Monitor abuse complaints and spam reports.
- Maintain good list hygiene by cleaning inactive emails.
Using services like Mystrika for email warmup and monitoring can also improve inboxing and prevent blacklisting headaches.
Managing Bounced and Undelivered Emails
It's essential to handle bounced emails instead of repeatedly sending to invalid addresses. Steps to take:
- Enable bounce notifications to get real-time failed delivery alerts.
- Add hooks to handle bounces - parse error messages and update status.
- Regularly review bounce reports to clean up bad addresses.
This avoids continued delivery attempts and preserves sender reputation.
Monitoring Inboxing Rates
Keep an eye on inbox placement rates to catch deliverability issues before they escalate.
- Use email tracking tools to check inbox vs. spam folder rates.
- Leverage inbox placement APIs to monitor ISP-level inbox rates.
- Act quickly if rates drop to diagnose and address potential problems.
Proactive monitoring ensures your important application emails reach the inbox. With some diligence, you can attain excellent deliverability.
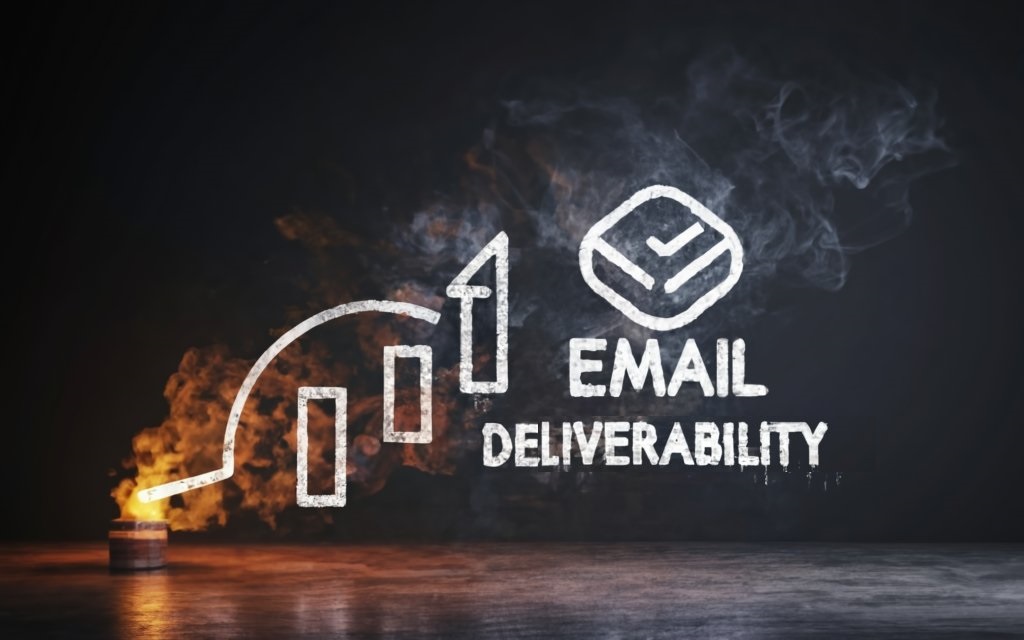
Optimizing Transactional Emails
Transactional emails like registration confirmations, purchase receipts, password resets etc. are a crucial touchpoint with users. Here are some tips to optimize these automated emails for engagement and conversions.
Customizing Registration and Password Reset Emails
Django sends rather plain registration and password reset emails by default. To make them more usable:
- Use HTML templates instead of just text content.
- Prepopulate username/email in reset links rather than making user look it up.
- Add custom sender name instead of just the email address.
- Include short intro copy highlighting next steps.
- Use custom email subjects like "Your account is ready!" instead of just "Password reset.
These small UX tweaks can positively impact activation and reset rates.
Adding Unsubscribe Links
Give users the option to opt-out of non-essential notification emails:
- Add unsubscribe links pointing to a view that flags the user.
- Have utility cron jobs to unsubscribe flagged users automatically.
- Periodically prune unsubscribed emails from lists.
This builds trust and gives users control.
A/B Testing Email Content
Try different email content variations to see what performs best:
- Vary the sender name, subject lines, calls to action.
- Test plain text vs HTML, short vs. long copy.
- Use open and click-through rates to identify the optimal version.
- Framework like Mailgun A/B Testing simplifies testing.
A data-driven optimization process maximizes engagement.
Personalizing Emails with Customer Data
Personalized emails deliver up to 6x higher transaction rates. Ways to personalize:
- Address users by first name in subject and body copy.
- Segment users based on preferences and purchase history.
- Recommend related products/content based on past activity.
- Trigger behavioral emails when users complete certain actions.
Leveraging customer data makes emails more relevant. With some thoughtful customization, you can really amplify the impact of transactional emails.
Integrating External Email Services
While Django provides basic email functionality out-of-the-box, integrating with external email services can enhance deliverability, analytics, and customization.
Setting up Mailgun with Django
Mailgun is a popular SMTP replacement that optimizes email delivery. To integrate it with Django:
- Sign up for a Mailgun account and add your sending domain.
- Install the Mailgun SDK for Python.
- Configure Mailgun credentials and domain in Django settings.
- Use the Mailgun API client to send messages.
Mailgun also provides useful email validation, tracking, analytics, templates etc.
Sending Emails via Amazon SES
For high-volume email, Amazon SES is a cost-effective SMTP alternative with good deliverability.
To set it up:
- Create an AWS account and verify your identity.
- Verify the sending domain in SES.
- Configure Django SMTP settings to use SES endpoints.
- Pass emails to Django's
send_mail()
using SES credentials.
SES integrates nicely with other AWS services like S3, Lambda, CloudWatch etc.
Importing Contacts from Mailchimp
To sync your Mailchimp audience with your Django app:
- Export the Mailchimp list as a CSV file.
- Write a script to import the contacts into your subscriber model.
- Schedule cron jobs to periodically sync for updated subscribers.
- Set up webhooks for real-time syncing.
This automates list management across tools.
Webhook Notifications on Email Events
Configure webhooks in Mailgun, SES or other services to post notifications to your app about email events like:
- Delivered emails
- Clicks and opens
- Unsubscribes
- Bounces or spam complaints
Consume these webhook notifications in Django to update stats, run workflows, log metrics etc.
Leveraging dedicated email services unlocks powerful functionality like deliverability optimization, analytics, automation workflows, event tracking etc. With the right integration approach, you can make your Django email capabilities enterprise-grade.
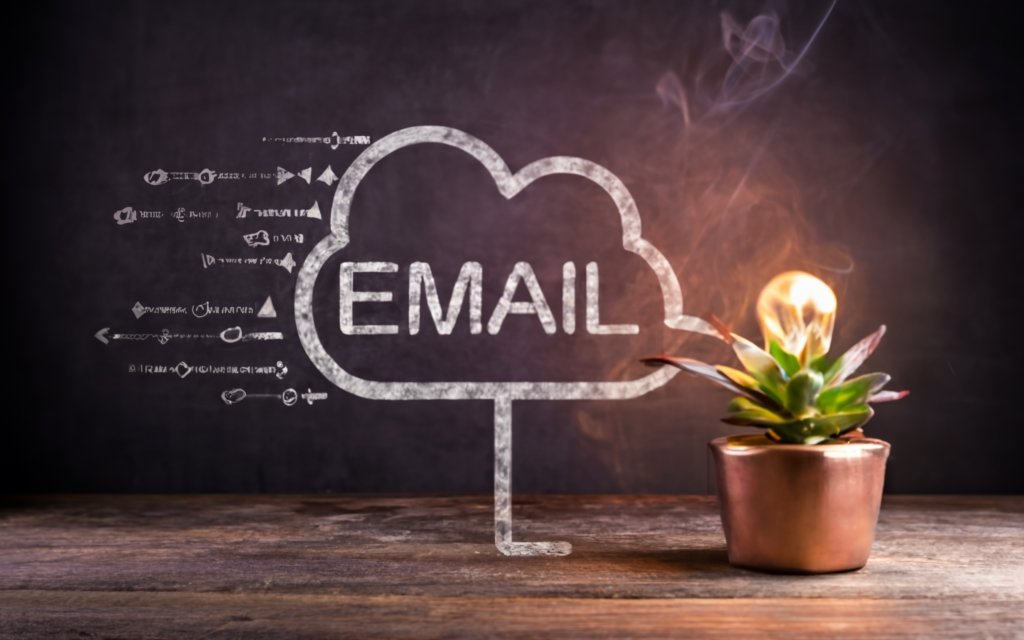
Building Custom Notifications and Alerts
Django's messages framework works great for one-off situational messages. But for recurring notifications like alerts, digests, reminders etc. a more robust system is required.
Here are some tips for building a custom notifications system:
Notification Models and APIs
The first step is modeling different notification types in the database, e.g.:
CLASS Notification(models.Model):
type = models.CharField(max_length=20)
recipient = models.ForeignKey(User)
message = models.TextField()
read = models.BooleanField(default=False)
sent_at = models.DateTimeField(auto_now_add=True)
Then build APIs to create notifications and mark them as read.
Queueing Notifications for Async Processing
Instead of sending notifications synchronously, add them to a queue for background processing using a library like Celery or Huey.
This avoids slowing down request-response cycle.
@shared_task
def send_notification(notification_id):
# Lookup notification and send
Delivering Notifications via Email, SMS, and Mobile Push
Support sending notifications via multiple channels like:
- SMS using a service like Twilio
- Mobile push notifications using Firebase Cloud Messaging
Segment users based on their preferred channel.
Allowing Users to Customize Notification Preferences
Let users select notification frequency, channels, and which event types to subscribe to. Model these as user preferences.
Some examples are:
- Daily/weekly digest vs. individual notifications
- Email vs. SMS delivery
- Subscribe/unsubscribe for order, promo, social notifications
This gives users control over their notification experience. By investing in a reusable notification infrastructure, you can streamline alerts and drive better user engagement.
Troubleshooting Email Issues
Even with the best email setup, issues can crop up that prevent messages from being sent and delivered properly. Here are some tips on debugging and fixing common email problems in Django.
Debugging SMTP Connectivity Problems
If the SMTP server is unreachable, no emails will be able to send. Some ways to troubleshoot connectivity issues:
- Try telnetting to the SMTP port to verify it's listening.
- Check for firewalls blocking the SMTP port traffic.
- Trace DNS resolution of the SMTP hostname.
- Verify SMTP credentials if authenticated delivery is enabled.
- Watch request logs to pinpoint any connection timeouts or access denials.
Getting basic connectivity is the first step towards sending emails.
Fixing Incorrect Email Headers
Improper headers like empty sender address or missing Message-ID can cause deliverability problems:
- Enable DEBUG mode during development to surface header errors.
- Set the proper domain, FROM address, and MESSAGE-ID configuration.
- Use libraries like django-email-extras to manage headers.
Proper headers improve deliverability and avoid issues with spam filters.
Understanding SMTP Status Codes
When troubleshooting, look at SMTP status codes in error messages - common ones include:
- 500s - Configuration issues on destination server.
- 400s - Temporary client errors like invalid syntax, exceeds quota, rejected by recipient domain.
- 300s - Success status for accepted message.
This gives clues on where the issue may lie.
Logging and Alerts for Email Errors
To detect problems early, log all 4xx and 5xx SMTP status codes. Set up monitoring and alerts on spikes in error rates.
Tools like Sentry can also aggregate and highlight email error trends. With diligent logging, testing, and monitoring, email errors can be identified and corrected quickly.
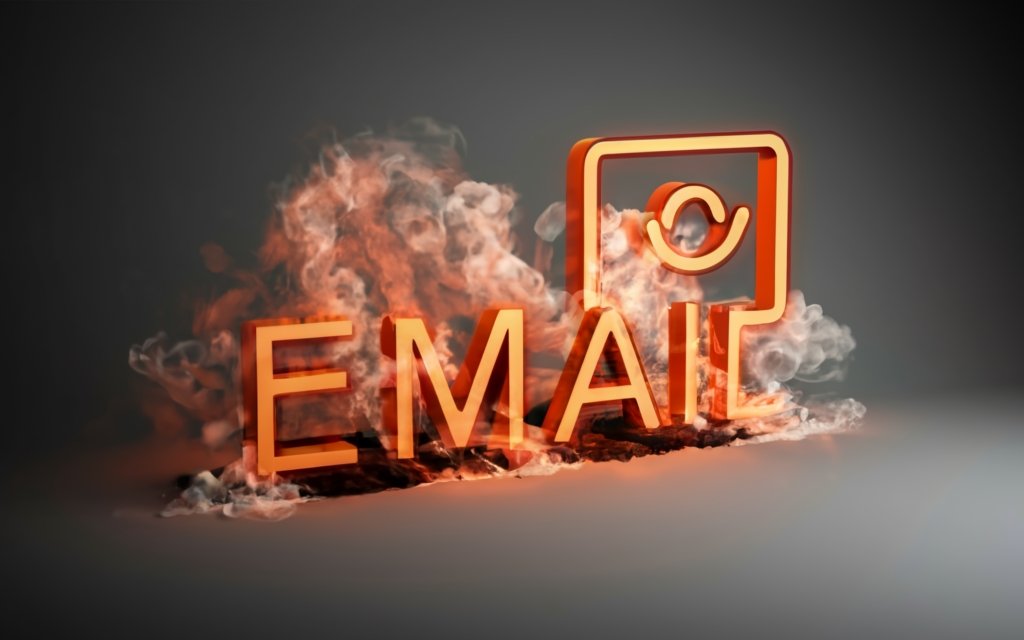
Testing and Monitoring Email Performance
Robust testing and monitoring helps catch email issues before they impact customers. Here are some key testing and performance tracking strategies for Django email.
Unit Testing Email Templates and Content
Leverage Django's test framework to unit test all aspects of email including:
- Rendering email templates with different contexts
- Validating generated HTML content
- Testing plain text alternate body content
- Ensuring proper subject lines
- Confirming recipient and sender addresses
This catches template bugs and incorrect content early.
Load Testing to Catch Deployment Issues
When deploying changes, load test by:
- Sending spikes of fake emails to validate connectivity
- Load testing queues and workers using locust, pytest-locust etc
- Monitoring for timeouts, errors, or high latency under load
- Tuning workers, connections, batches based on load test findings
This confirms the email system behaves under production load.
Uptime Monitoring for Email Infrastructure
Use uptime checks to monitor key infrastructure like:
- SMTP servers and webhooks
- Queue workers
- Supporting services like S3, database
- External providers like Mailgun, Postmark
Get alerts if any component goes down impacting email capabilities.
Analyzing Email Analytics and Reports
Leverage email tracking tools like Mailgun, Sentry, Postmark to:
- Check email delivery rates, retries, bounces
- Spot trends in failed/flagged emails
- Optimize topics, sender, content that resonates best
- Identify potential reputation issues early
Ongoing analysis provides actionable insights into improving email performance. With a combination of testing, monitoring, and analytics you can keep email delivery optimized and reliable.
Scaling High-Volume Email Delivery
Django's email capabilities work great out of the box, but need some tweaking to handle large volumes. Here are some tips for scaling email delivery:
Upgrading to Dedicated SMTP Services
For high throughput, use dedicated SMTP services like Amazon SES, Mailgun, or Postmark instead of your own SMTP server or ESPs like Gmail.
Benefits include:
- Higher sending limits of millions of emails per month
- Lower deliverability issues with dedicated IP reputation
- Support for bulk sending, templating, analytics etc.
Optimizing Queries for High-Performance Queues
Use database-backed queues like Celery with Redis or RDBMS. Optimize query performance:
- Use worker chunking to fetch and process messages in batches instead of one-by-one.
- Prefetch related data in bulk to avoid n+1 queries.
- Add database indexes on queue metadata fields for faster filtering.
- Cache frequently used query results.
A fast and efficient queue improves throughput.
Running Queue Workers on Multiple Machines
Distribute queue workers across multiple machines, like Kubernetes pods, to increase processing capacity.
Strategies like using separate queues or workers for high vs. low priority jobs also helps.
Caching Content for Frequently Reused Emails
Leverage in-memory caches like Memcached to store rendered email content, especially for bulk emails.
This avoids expensive repeated template rendering for each recipient.
Load test your setup thoroughly to catch bottlenecks early. With some strategic optimizations, you can comfortably scale Django email delivery for high loads.
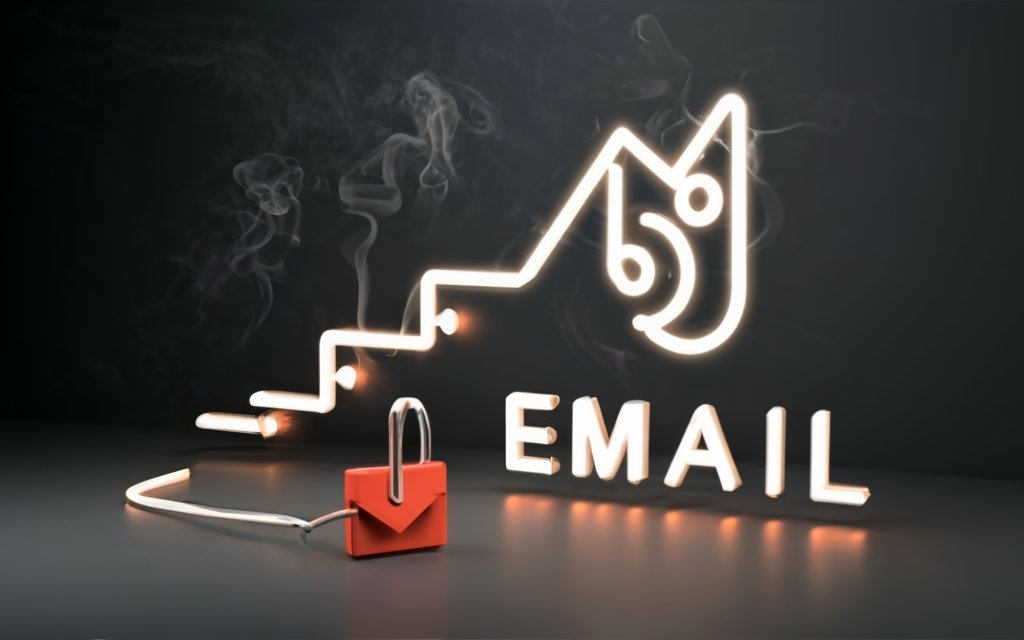
Summary
Here are the key takeaways from this comprehensive guide on messages, emails, and notifications in Django:
- Django provides a flexible built-in messaging framework that can be used for situational messages, alerts, notifications, and more.
- Validating and normalizing email addresses is crucial before sending emails. Use Django's EmailField, write custom validators or leverage third-party email validation APIs for robust validation.
- Configure SMTP properly and leverage libraries like EmailMessage and EmailMultiAlternatives for sending emails with attachments, templates etc. Use services like Mailgun, SES, Postmark instead of SMTP for deliverability.
- Follow best practices like warming up IP addresses, monitoring blacklists/reputation, handling bounces, and tracking inboxing rates to ensure emails reach the inbox.
- Optimize transactional emails by customizing content, adding unsubscribe links, A/B testing, and personalizing with customer data.
- Integrate external services like Mailgun, SES, and Mailchimp via APIs and webhooks for greater customization, deliverability, analytics, and automation.
- Build reusable notification models, APIs, queues, and allow delivery via multiple channels like email, SMS, push etc. Give users controls over their notification preferences.
- Set up diligent error monitoring, logging, testing, and analytics to catch email issues early and optimize performance over time.
- Scale email delivery by upgrading infrastructure, optimizing queues, implementing caching, and load testing thoroughly before launching.
With these tips and best practices, you can handle all your messaging, email, and notification needs reliably in Django.
Frequently Asked Questions
Here are some common questions about messages, emails, and notifications in Django:
How do I display a one-time message in Django templates?
Use the built-in messages framework. In your view, call messages.success(request, 'Message text')
etc. to set messages. Then in templates, check if messages exist and iterate through them to display.
What's the best way to validate email addresses in Django?
Use Django's EmailField and EmailValidator if you just need a basic regex check. For more robust validation, write a custom validator or use a dedicated API like AbstractAPI's email validation API.
How can I customize registration and password reset emails in Django?
Override the default templates for these emails in your project's templates/registration/ folder. Customize the subject, add some copy, brand it, and leverage the context like user's name.
What is the best way to add unsubscribe links to emails?
Create an Unsubscribe
model to track users who want to opt-out. When rendering emails, check if the recipient user has opted out and display unsubscribe links accordingly. Have a view to process unsubscribe requests.
Should I use Django's SMTP server or external providers for sending emails?
External SMTP providers like Mailgun, Amazon SES, Postmark etc. are recommended for better deliverability, analytics, and scalability. But Django's SMTP is easier to get started with.
How do I integrate my Django app with transactional email providers?
Most have client libraries or APIs to integrate with. For example, to use Mailgun, install their Python SDK, configure API credentials, and use their mail.send()
API to send Django emails.
What are some good strategies for scaling high-volume email delivery?
Upgrading to dedicated SMTP services, optimizing queue performance, distributing queue workers, caching reused content, upgrading server capacity, load testing, etc. can help scale Django email.
How can I be notified of email events like bounces or opens?
Many ESPs provide webhooks to get notifications for events. Handle these webhook requests in a view to process events like marking bounces, updating open stats etc.
How do I set up monitoring and reports for email performance?
Leverage provider tools like Mailgun or Sentry for monitoring and reports. Log email errors to monitor failure rates. Track open rates, click rates, and unsubscribes to optimize content.