Tired of clumsy authentication code cluttering up your sleek Ruby on Rails app? Devise is here to save the day! Learn how to painlessly add rock-solid user signup, login, social Oauth, and other authentication goodies with this comprehensive Devise guide. We’ll explore how this gem works its magic under the hood, customize it seven ways to Sunday, handle scaling and security, and debug even the trickiest issues. Come kick user authentication to the curb, the Rails way!
Understanding Devise Basics
Devise is one of the most widely used authentication solutions for Ruby on Rails applications. With over 23,000 stars on GitHub and usage in over 522,000 public repositories, Devise has become the go-to gem for implementing user sign-up, login, and account management in Rails apps.
But what exactly is Devise, and why has it become so popular? Let’s break it down.
What is Devise and why use it?
Devise is an authentication framework that provides a full-featured and flexible solution for user authentication in Rails. It is designed on Warden and offers a clean, organized way to implement essentials like:
- User login/logout
- Account registration
- Password encryption and resetting
- Session management
- Account confirmation via email
- OmniAuth integration for social login
Devise wraps all these complex authentication tasks into easy-to-use Rails engines and controllers. You don’t have to build user authentication from scratch or handle edge cases like forgotten passwords yourself.
Simply install the Devise gem, generate a Devise user model, and configure any modules you need – Devise handles the controllers, routes, and views automatically in a Rails-friendly way.
Some key benefits of using Devise include:
- Time savings – Devise eliminates the tedious authentication work so you can focus on your app.
- Cleaner code – Devise encapsulates authentication logic in one place rather than scattering it.
- Standardized – Following Devise conventions makes your app familiar to other developers.
- Flexible – Devise offers various modules and customization so you only use what you need.
- Well-tested – With widespread use in production apps, Devise is a stable and robust solution.
For these reasons, Devise has become the default choice for authentication in most Rails apps today.
Core features and benefits of Devise
To understand Devise better, let’s look at some of its core modules and features:
- Database Authenticatable – Handles password authentication and encryption using BCrypt.
- OmniAuthable – Adds support for social and OAuth login via the OmniAuth gem.
- Confirmable – Sends confirmation emails and checks account confirmation status.
- Recoverable– Resets passwords and sends reset instructions via email.
- Registerable – Handles user registration, editing, deletion of accounts.
- Rememberable – Manages persistent login sessions via browser cookies.
- Trackable – Tracks and stores sign in counts, timestamps, and IP addresses.
- Timeoutable – Sets configurable timeouts for user sessions.
- Validatable – Adds email and password validations.
- Lockable – Locks accounts after a specified number of failed sign-in attempts.
Devise includes all this out-of-the-box, customizable via simple Rails generators. You don’t have to build any of it yourself!
Beyond these modules, Devise follows Rails conventions and provides controller helpers, routes, and views that integrate seamlessly. Migrating between Rails versions is easy as Devise fully supports the latest Rails releases.
When not to use Devise as a Rails beginner
While Devise is extremely useful, it does add a layer of “magic” that may confuse Rails beginners. The README itself notes:
If you are building your first Rails application, we recommend you do not use Devise. Devise requires a good understanding of the Rails Framework.
As a beginner, it’s better to build authentication yourself following a tutorial to fully grasp concepts like:
- How sessions and cookies function
- How to securely store passwords
- How RESTful architecture handles login requests
- Where authentication logic should live in MVC
Once you have this foundational knowledge, Devise will be much easier to use productively. But for your first Rails app, go ahead and try rolling your own authentication – you’ll learn a lot!
The key is that Devise provides powerful shortcuts to avoid authentication boilerplate. Fully understanding authentication first will make you appreciate Devise much more!
So in summary:
- Devise is the most popular and flexible authentication solution for Rails.
- It provides a full-featured set of authentication functionality out-of-the-box.
- Using Devise saves time and avoids messy one-off authentication logic.
- But Rails beginners may benefit from learning authentication basics before utilizing Devise.
Now that you have a high-level understanding of Devise, its features, and when to use it, let’s move on to actually implementing it in a Rails application next.
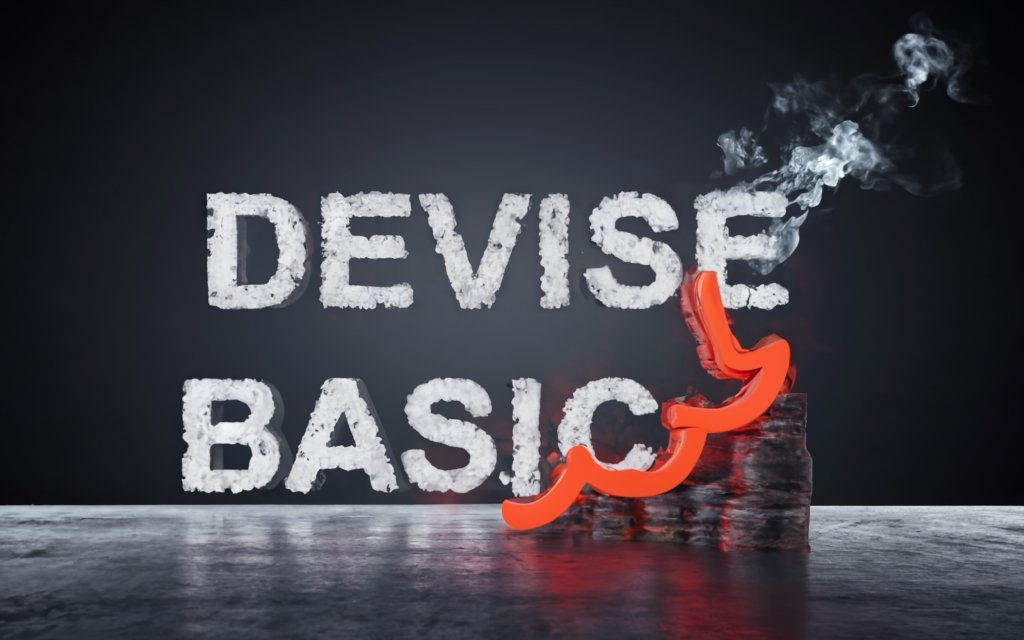
Getting Started with Devise
Ready to dive in and implement Devise? In this section, we’ll walk through installing Devise and adding basic sign-up and login to a fresh Rails application.
Follow along to get hands-on experience with a Devise foundation you can build on later.
Installing and configuring Devise
Let’s start by initializing a new Rails 7 app:
rails new my_app
First, add the Devise gem to your Gemfile:
gem 'devise'
Run bundle install
, then use the generator to install Devise:
rails generate devise:install
This creates the Devise initializer at config/initializers/devise.rb
with instructions at the top. You’ll need to set the default URL options for Action Mailer based on your environment.
For development, add in development.rb
:
config.action_mailer.default_url_options = { host: 'localhost', port: 3000 }
No need to set anything for test.rb
.
For production, include your actual hostname:
config.action_mailer.default_url_options = { host: 'your-app.com' }
This allows Devise to form proper links in emails.
Next, you’ll need to add Devise flash messages to app/views/layouts/application.html.erb
:
<p class="notice"><%= notice %></p>
<p class="alert"><%= alert %></p>
These messages will display signup/login statuses.
That covers initial Devise configuration – let’s create our user model next!
Generating a Devise user model
Devise needs a model for authentication. The standard naming convention is to call this model User
:
rails generate devise User
This generates the User
model, a migration to create the users
table, and inserts a devise_for :users
route in routes.rb
.
Before we migrate, let’s peek at the migration file Devise created at db/migrate/<timestamp>_devise_create_users.rb
:
class DeviseCreateUsers < ActiveRecord::Migration[7.0]
def change
create_table :users do |t|
## Database authenticatable
t.string :email, null: false, default: ""
t.string :encrypted_password, null: false, default: ""
# ...
end
end
end
We can see Devise has created email and encrypted password fields, along with null constraints and default values, for authentication.
Now run the migration:
rails db:migrate
Our users
table is now ready!
Adding Devise controllers and routes
Remember the devise_for :users
route added to routes.rb
? This line actually creates many authentication routes automatically:
new_user_session_path => /users/sign_in
destroy_user_session_path => /users/sign_out
new_user_password_path => /users/password/new
# ...
You don’t need to manually define each route for signing in, out, password reset, etc. Devise handles this “magic” for you.
These routes will point to the Devise controllers in app/controllers/users/
for handling these actions. Again, you don’t need to write these controllers!
Linking views to Devise pages
We now have everything in place to start building views. Let’s add a simple home page at app/views/home/index.html.erb
:
<h1>Home</h1>
And link to it as the root route in routes.rb
:
“`ruby
root ‘home#index’
Now add links for signing in and signing up:
erb
<%= link_to “Sign in”, new_user_session_path %>
<%= link_to “Sign up”, new_user_registration_path %>
These use the path helpers Devise created. Visiting the root URL and clicking “Sign up” will now land on the Devise-powered sign up page!
Sign up with a test email and password. You’ll automatically be signed in and redirected back home.
Recap and next steps
That was a whirlwind Devise intro! We:
- Installed and configured Devise with flash messages and mailers
- Generated a Devise User model with secure password handling
- Added Devise controllers and routes automatically
- Linked sign up/in pages to our home page
We now have a solid Devise foundation. Later we can explore:
- Devise custom controllers, routes, and views
- Adding authentication helpers
- Authentication via OmniAuth
- Confirmable, recoverable, and other modules
- Admin roles and permissions
The world of user authentication possibilities is now open!
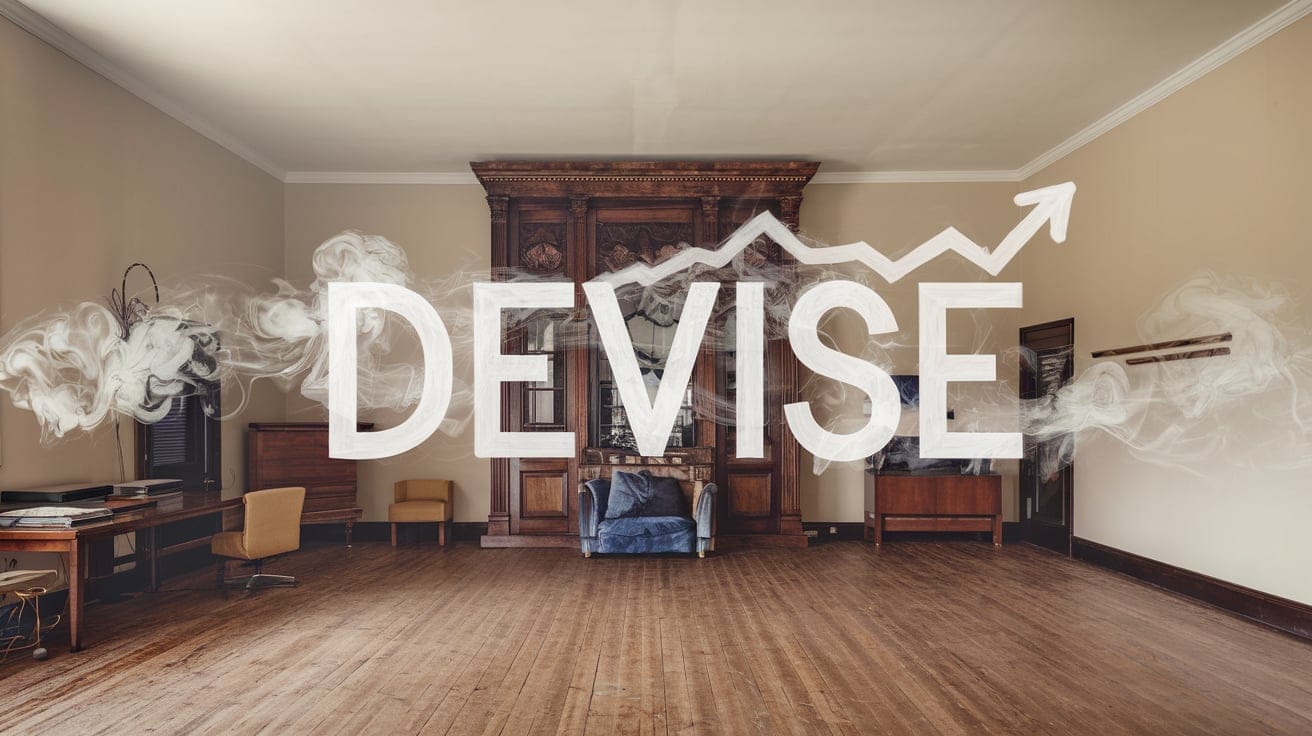
Using Devise Features
Database authentication strategies
-Overview of how Devise handles authentication against the database
-Examples of database_authenticatable module usage
-BCrypt encryption specifics
-Customizing authentication strategies
Password encryption and validation
-Devise’s use of BCrypt for password hashing
-Configuring password complexity rules
-Minimum password length, allowing special characters
-Length and digest options for encryption
-Adding custom validations
Account confirmation emails
-Using Devise’s confirmable module
-Customizing confirmation instructions
-Setting after_confirmation callback
-Handling reconfirmation and unconfirmed accounts
-Adding confirmable to an existing model
Forgot password and account recovery
-Enabling recoverable module
-Password reset token security and storage
-Customizing reset emails and instructions
-Setting password reset timeouts
-Showing status after reset request
Rememberable – using cookies for user sessions
-How rememberable uses cookies to persist sessions
-Cookie expiration configurations
-Refreshing remember token on activity
-Disabling rememberable while allowing sign in
-Additional browser session considerations
Tracking login counts, timestamps, IPs
-Using Devise’s trackable module
-Storing and accessing tracked login data
-IP logging concerns and handling GDPR/privacy regulations
-Tracking timestamps of last sign-in and current sign-in
-Beware sign-in count issues if using OmniAuth
Setting login timeouts
-Idle vs absolute timeout configurations
-Possible race condition and mitigations
-Testing for timeout behavior
-Using timeouts to auto-logout inactive sessions
Locking user accounts after failed attempts
-Enabling Devise’s lockable module
-Setting number of attempts and lock timeframe
-Unlock via email or after time period
-Revealing info about locked state
-Lock strategy tradeoffs and alternatives
OmniAuth for social login
-Overview of integrating OmniAuth with Devise
-Setup and configuration steps
-Common providers like Facebook, Twitter, Google
-Creating custom providers
-Handling provider authentication callbacks
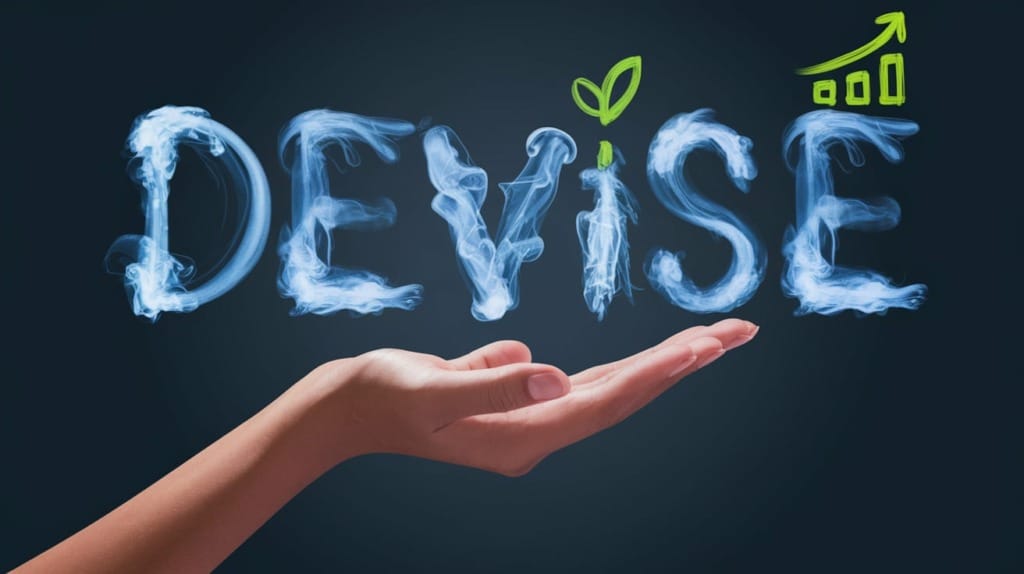
Advanced Devise Customization
Once you have Devise installed and the basics working, eventually you’ll need to customize it to suit your application’s needs. Devise offers multiple ways to tailor authentication to your requirements.
Let’s explore some of the most common Devise customizations including controllers, routes, views, mailers, jobs, and debugging.
Overriding Devise controllers and routes
Devise generates controllers like SessionsController
to handle authentication-related actions. You can override these controllers by creating customized versions.
First, generate a controller while telling Devise not to make it:
rails generate devise:controllers users -c sessions
This creates the controller class Users::SessionsController
for you to customize.
For example, you may want to direct users to a custom page after signing in. Override the create
action:
def create
super do |resource|
redirect_to home_dashboard_path
end
end
Call super
to retain default behavior, then add a custom redirect_to
.
To use this controller, tell the Devise route to use a custom controller:
devise_for :users, controllers: { sessions: 'users/sessions' }
You can also override routes like registrations
and passwords
similarly.
Customizing Devise views
To override Devise’s default views, run:
rails generate devise:views
This copies all views to your app for editing. For example, you can customize the sign up view at app/views/devise/registrations/new.html.erb
.
Devise uses a devise/
directory so it can control the default views. But you can freely modify copies in app/views
.
Adding custom mailers
Devise uses ActionMailer to send emails for confirmation and password resetting. You can generate a custom mailer:
rails generate devise:mailer User
This creates a UserMailer
you can customize at app/mailers/user_mailer.rb
.
Be sure to configure Devise to use the custom mailer in initializer/devise.rb
:
config.mailer = 'UserMailer'
Now UserMailer will handle Devise emails.
Integrating with background jobs
To send Devise emails asynchronously via ActiveJob:
class User < ApplicationRecord
devise :database_authenticatable,
:registerable,
:confirmable,
:recoverable,
:rememberable,
:validatable,
:trackable
# Deliver Devise emails with ActiveJob
def send_devise_notification(notification, *args)
devise_mailer.send(notification, self, *args).deliver_later
end
end
Now confirmations, password resets, and other communications will happen in the background.
Debugging issues with Warden
Since Devise is built on Warden, many issues boil down to Warden misbehaving.
A common problem is the Warden::NotAuthenticated
exception. To debug:
- Reproduce the error and grab the Warden message.
- Set
config.debug_warden = true
indevise.rb
. - Trigger the error again and inspect the Rails log.
- Turn debug back off in
production.rb
.
This can reveal useful information like misconfigured scope or unauthenticated states.
For other Warden issues, it may help to:
- Call
warden.authenticate!
manually and inspect the response. - Check whether the user is authenticated via
warden.authenticated?(scope)
- Utilize Warden callbacks like
after_failed_fetch
andafter_fetch
- Use
warden.on_next_request
if authentication state seems unclear
With tricky problems, Warden debugging is your best friend. Consult the Warden wiki for advanced debugging docs.
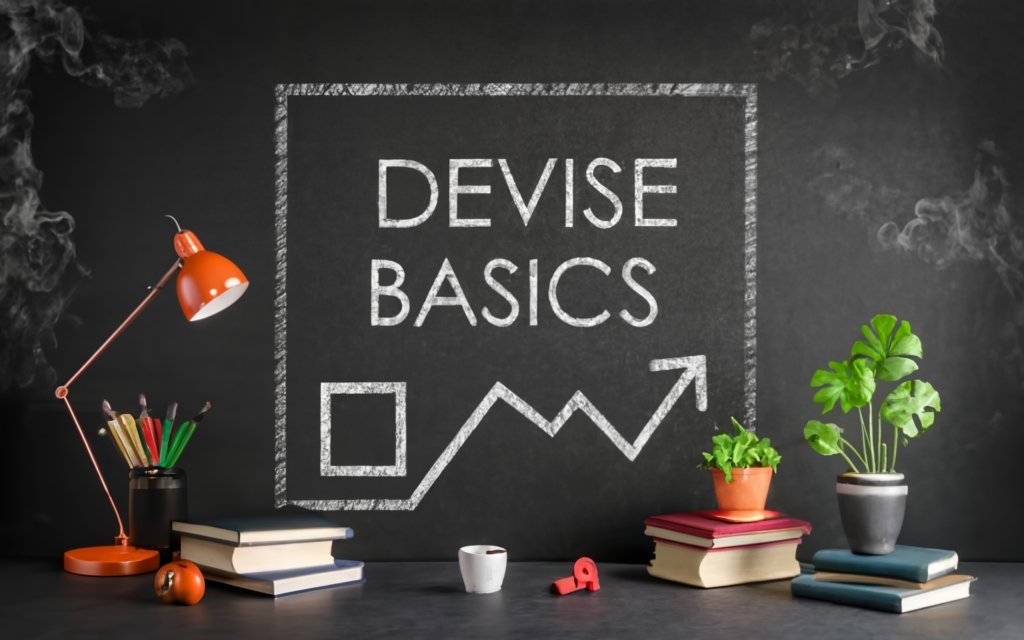
Resetting the Database in Rails
Dealing with a messy development database that has drifted from schema? Starting fresh with accurate data can work wonders. Here’s when and how to reset your Rails database cleanly.
When and why database reset is needed
After adding and removing migrations, sometimes the database schema and actual structure get out of sync.
Common scenarios where a reset helps:
- Mystery data appears in production from old migrations
db:migrate
fails saying the table already exists- Team members have conflicting migrations that won’t apply cleanly
- Need to re-seed sample data from scratch
- Tests fail because existing data causes conflicts
Resetting lets you:
- Synchronize the schema from
schema.rb
- Run all migrations again in order
- Clear out old or duplicate data
Starting with a fresh database can fix many bizarre issues that accumulate over time.
Key reset tasks – db:migrate, db:rollback, db:reset
Rails provides several rake tasks to reset the database:
db:migrate
– Runs any new migrations to bring the schema up to date.db:rollback
– Reverts the last migration by runningdown
method.db:reset
– Drops, recreates, migrates, and seeds the database completely.
Let’s explore these tasks in more detail:
db:migrate
This task runs any pending migrations that haven’t been applied yet. It brings the schema to the latest version.
rake db:migrate
You can also target a specific version:
rake db:migrate VERSION=20080906120000
db:rollback
This task reverts the last migration by running its down
method.
rake db:rollback
You can roll back multiple migrations by passing STEP
:
rake db:rollback STEP=3
db:reset
This task drops the entire database, recreates it, runs all migrations, and seeds it.
rake db:reset
db:reset
is the sledgehammer – use it when you really need a completely fresh database.
Seeding sample data after reset
Resetting will empty the database, deleting any existing data.
To populate the empty database with sample data, use the db:seed
task:
# db/seeds.rb
Product.create(name: "Widget", price: 25)
Product.create(name: "Gadget", price: 50)
Now when you run:
rake db:seed
It will create the sample products in the database.
Some tips:
- Check for existing data before inserting to avoid duplicates.
- Write idempotent seeds that can re-run safely.
- Use
db:setup
to migrate, seed, and reset in one step.
Caveats and downsides of reset
Database resets are not without downsides:
- Any existing real data will be deleted.
- Resetting can mask issues that should be fixed properly.
- Migrations may add data that can’t be easily recreated.
- It interrupts and blocks other devs sharing the database.
- Extra load on the database server to recreate & migrate.
- Won’t fix bad migrations that have already run.
So when should you reset? Here are a few best practices:
- Only reset in development, never production data.
- Reset early in a project before much data accumulates.
- Reset frequently in early dev to avoid large migrations.
- Communicate with team members before doing a reset.
- Reset as needed in CI/test environments.
With discipline, occasional resets can keep a project’s database healthy and migrations running smoothly.
Troubleshooting Errors and Issues
Despite the best laid plans, things can still go wrong. Here are some common Devise errors and how to fix them.
Fixing “inbox task is not valid for user” in Gmail
If you get the error “Inbox task is not valid for this user” in Gmail when clicking confirmation links from Devise, a few things could be going on:
- Check that the Gmail account is not restricted. School/work accounts may have limitations. Try a regular Gmail address.
- Make sure accessing Gmail from the same location/IP as the confirmation email was sent from. Gmail sometimes blocks different regions.
- Double check the confirmation link is properly formatted and contains the user’s unique token.
- Log out and back into Gmail. There is an edge case where Gmail gets confused on identity.
- Try opening the link in an incognito window. Extensions like AdBlock can interfere.
- As a last resort, generate a new confirmation token and email for the user.
With a bit of trial and error, the exact cause can usually be isolated.
Authentication routing and missing method errors
Here are some common routing-related issues:
Getting a No route matches
error for new_user_session_path
? Double check devise_for :users
exists in routes.rb
.
Seeing an undefined method
users_url` error? Add this to the Devise initializer:
```ruby
config.navigational_formats = ['/', :html, :turbo_stream]
The method `current_user` unknown? Make sure to use the Devise `@current_user` variable, not the default Rails `current_user` helper. Getting an error in `ApplicationController` like `undefined method authenticate_user!`?
Be sure to add `before_action :authenticate_user!` in controllers that require authentication.
Ensuring database schema loads properly If migrations are stalling or failing with schema issues:
– Delete the `db/schema.rb` file and recreate with `rake db:schema:dump`.
– Reset the database with `rake db:reset` to start from a clean slate.
– Check for duplicate or obsolete migrations causing conflicts. The schema may be out of sync.
– Enable SQL logging to inspect failing migration statements:
ruby
class Application < Rails::Application
config.active_record.logger = Logger.new(STDOUT)
end
```
Then you can pinpoint any incorrect SQL.
Debugging stalled or failed migrations
If a migration gets stuck midway and keeps failing:
- Roll back the migration with
db:rollback
. - Edit the migration to fix errors, then run again.
- Split large migrations into smaller pieces for easier debugging.
- Print debugging statements and inspect the logs to isolate issues.
- Create a new migration to undo/redo changes if rolling back didn’t fix it.
- In a worst case scenario, manually edit the database or create SQL dumps.
Don’t forget to delete any rows added halfway by a failed migration to avoid data issues.
Hopefully these tips will help get things running smoothly again. But Devise issues can often come down to subtle configuration problems or edge cases. If stuck, don’t hesitate to search the repos, ask on StackOverflow, or reach out to the Devise community.
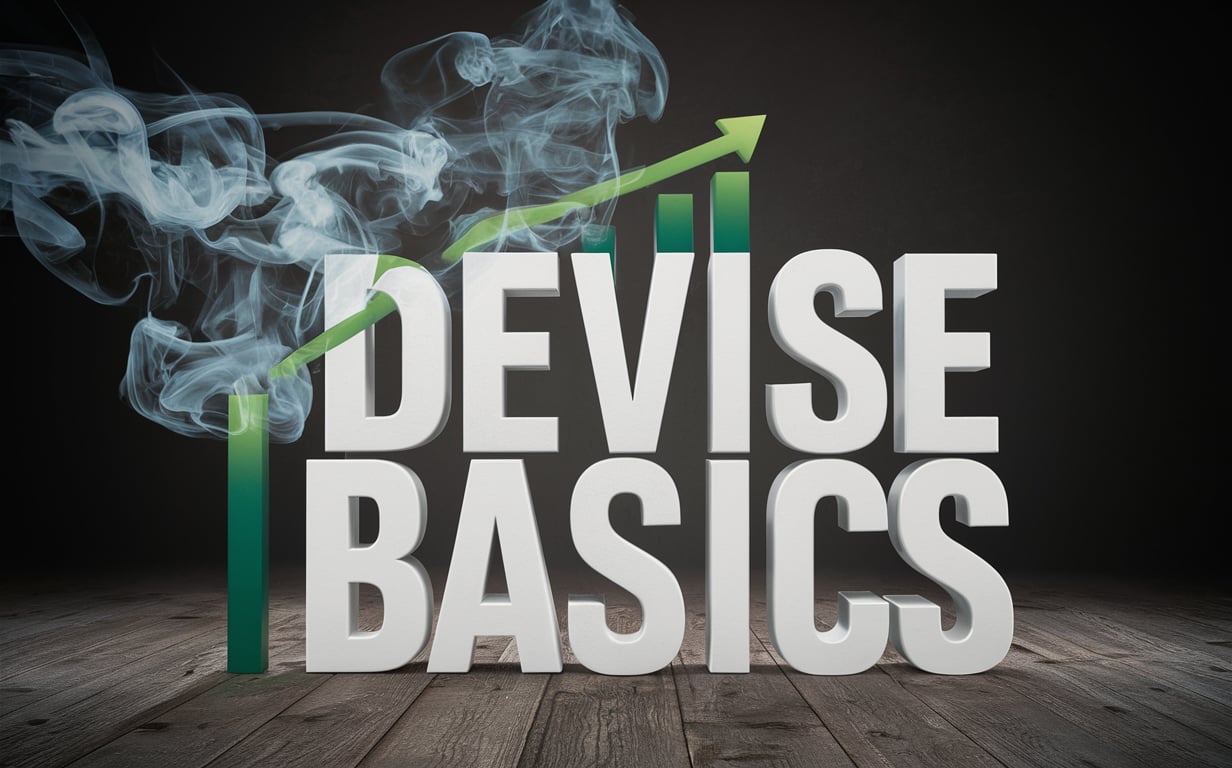
Best Practices for Production-Ready Authentication
You’ve mastered Devise basics, but deploying authentication to production brings new concerns like security, performance, and scale. Here are some tips for bulletproofing your app.
Additional security considerations
A few extra precautions to take:
- Use SSL/HTTPS everywhere – no plain HTTP authentication.
- Enable Rails’ CSRF protection to prevent forgery attacks.
- Strong password policies – enforce minimum length, complexity, expiration, etc.
- Regularly audit dependencies for vulnerabilities with tools like Bundler Audit.
- Consider flagging or locking accounts after repeated failed sign-in attempts.
- Provide a way to report security issues and be responsive.
- Implement encryption at rest for passwords: don’t just hash, but use a pepper key.
- Require reconfirmation if a user’s email or other details change.
Think through the weak points and keep security top of mind.
Performance optimization tips
Here are some Devise optimizations for snappier auth:
- Enable caching of Devise helpers like
authenticated?
andsigned_in?
via Redis or Memcached. - Use eager loading associations on the User model to reduce queries.
- Set a proper index on your User model’s email field for faster lookup.
- Tweak the cost factor for
BCrypt::Password
to balance security and speed. - Lazy load non-critical Devise modules like
:confirmable
on the happy path. - Avoid N+1 queries when fetching user roles, permissions, etc.
Review slow requests and make auth calls optimized.
Handling customer authentication at scale
For large user bases, a few scalability considerations:
- Introduce request caching of Devise requests via your CDN.
- Rate limit login attempts per IP to prevent brute force attacks.
- Partition your User database by cohort for faster querying.
- Run a regular audit process to deactivate old unused accounts.
- Shard your database across multiple nodes for read/write scaling.
- Plan for failover – consider implementing multi-region redundancy for uptime.
- Handle spikes in traffic by auto-scaling your dynos or nodes.
With size comes complexity – plan ahead to scale smoothly.
Integrating monitoring and analytics
Visibility into auth operations is crucial:
- Use NewRelic, Skylight, or Scout to monitor Devise performance.
- Integrate logging with solutions like LogRocket or Sentry.
- Collect metrics on login success/failures, new registrations, etc.
- Implement real user monitoring to track authentication flows.
- Set alerts around error rates, latency, or abnormal traffic.
- Audit logs regularly for suspicious activity – failed logins, brute force attacks.
- Analyze trends across signup sources – email vs social.
Telemetry around authentication helps spot issues early and optimize workflows.
By following security best practices, optimizing performance, planning for scale, and implementing monitoring, you can feel confident trusting Devise with your production authentication needs.
With an adaptable framework like Devise and some diligence, your app’s auth can scale to millions of users without a hitch!
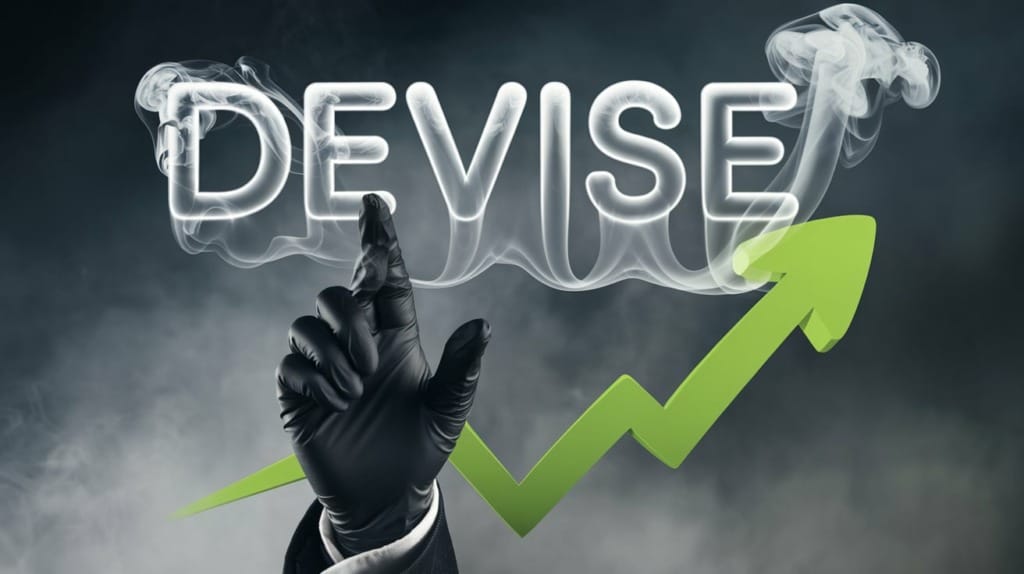
Key Takeaways
After reading this extensive guide, you should have a firm grasp on implementing robust authentication with Devise in Ruby on Rails.
Let’s recap the key points:
- Devise is the most flexible and fully-featured authentication solution for Rails. It handles everything from encryption to mailers.
- Carefully consider if Devise is appropriate for total beginners – start simple before introducing magic.
- Follow best practices for installation and configuration – set default URLs, add flash messages, etc.
- Easily generate Devise user models with critical secure password handling.
- Customize controllers, routes, views, mailers and more to tailor Devise to your app.
- Enable modules like Rememberable and OmniAuth incrementally as needed.
- Reset your development database occasionally to clear out stale migrations or data.
- Debug issues by enabling Warden logging, checking routing errors, and inspecting logs.
- In production, secure accounts with encryption, monitor analytics, and plan for scale.
- Leverage Devise callbacks and hooks to deeply integrate authentication across your app.
With Devise’s flexibility, you can add just the features you need today while keeping the door open to continue extending as your authentication needs evolve.
Now you have all the tools to implement feature-rich authentication using this Ruby on Rails standard. The only limit is your imagination – happy coding!
Frequently Asked Questions
How do I add new fields like username to the Devise user model?
Use a migration to add columns to the users table, then expose them on the model using attr_accessor
. Be sure to permit them in the Devise registrations_controller
too.
Why are my password resets or confirmations not sending emails?
Double check that you have configured SMTP and default_url_options correctly in the Devise initializer and environments files.
How can I change the default Devise routes?
You can customize the path names by passing options like path_names
to devise_for
in routes.rb
.
Why are my Devise views not overriden when I modify them?
Be sure to generate Devise views first using the rails generate devise:views
command so the files exist in your app for editing.
How do I authenticate an API request using Devise?
Use the Devise::JWT::RevocationStrategies::Allowlist
strategy to authenticate API requests using JSON Web Tokens instead of cookies.
Can I use Devise for social login via Facebook/Twitter/Google?
Yes, Devise integrates nicely with OmniAuth to support authentication via social platforms using OAuth.
How can I define different roles and permissions for users?
Use a permissions gem like Pundit or Rolify in conjunction with Devise to define granular user roles and abilities.
What are best practices for overriding Devise controllers?
Create custom controllers by running rails g devise:controllers [scope] -c [controller]
and be sure to tell Devise to use it in routes.
How do I troubleshoot problems with Devise and Warden?
Enable Warden debugging, watch the logs, check the scope, and use hooks like after_failed_fetch
to isolate issues.
What are some optimizations for improving Devise performance?
Enable caching of helpers, eager load associations, set proper indexes, and monitor with tools like Skylight.